Flutter表情符号管理插件gugor_emoji的使用
Flutter表情符号管理插件gugor_emoji的使用
gugor_emoji简介
gugor_emoji
是一个用于在 Flutter 应用程序中管理和选择表情符号的插件。它提供了丰富的功能,包括自定义视图、皮肤色调支持等。
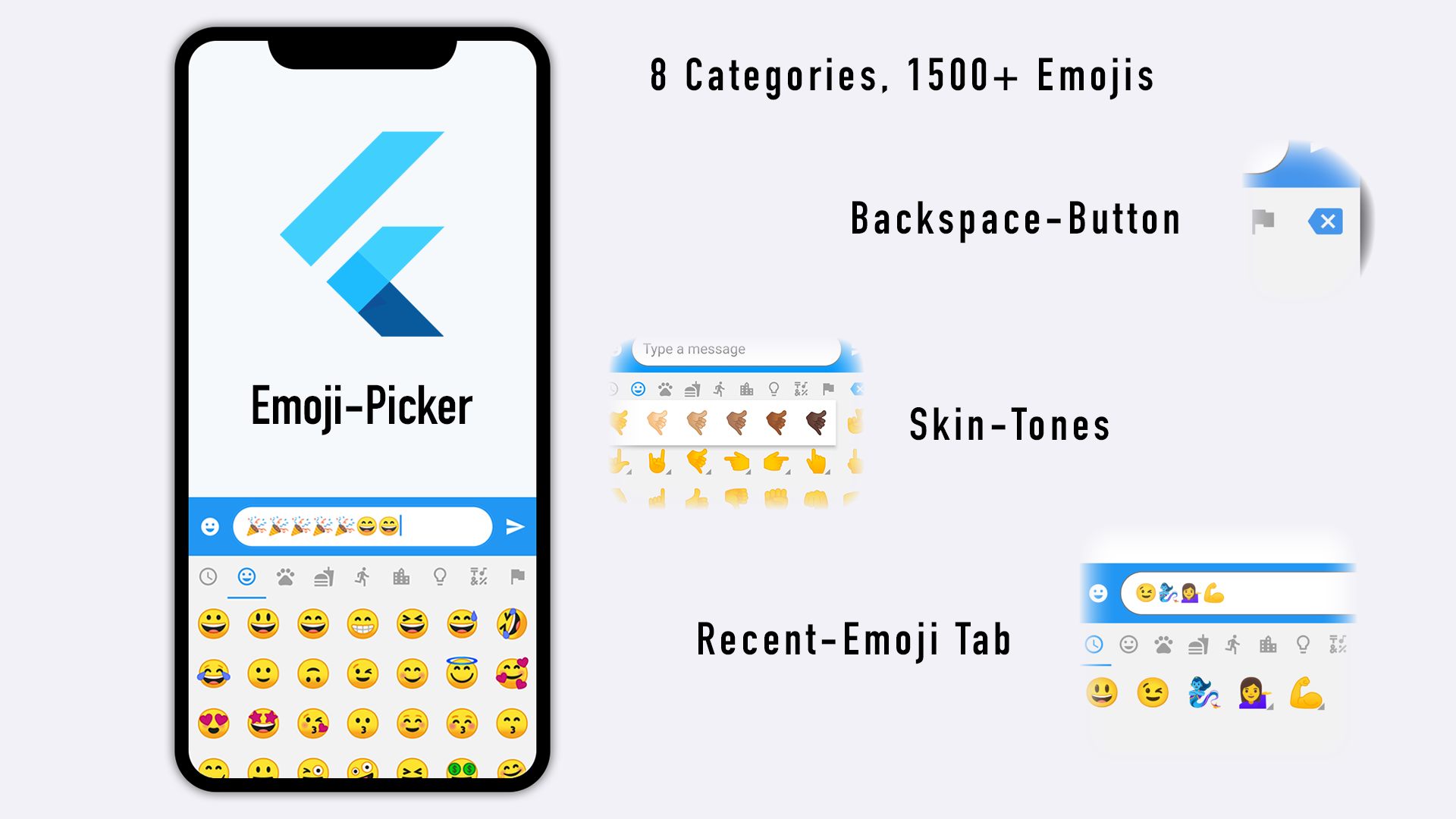
关键特性
- 轻量级包
- 快速加载
- 空安全
- 完全可定制
- Material Design 和 Cupertino 模式
- 过滤无法显示的表情符号(仅限 Android)
- 可选的最近使用的表情符号标签
- 支持皮肤色调
开始使用
要开始使用 gugor_emoji
插件,你需要首先在你的项目中添加依赖项。你可以在 pubspec.yaml
文件中添加以下依赖:
dependencies:
gugor_emoji: ^x.x.x
然后运行 flutter pub get
来获取新的依赖项。
基本示例
下面是一个基本的使用示例,展示了如何在应用程序中集成 gugor_emoji
插件。
import 'dart:io';
import 'package:gugor_emoji/emoji_picker_flutter.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
/// 示例代码
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
final TextEditingController _controller = TextEditingController();
bool emojiShowing = false;
_onEmojiSelected(Emoji emoji) {
_controller
..text += emoji.emoji
..selection = TextSelection.fromPosition(
TextPosition(offset: _controller.text.length));
}
_onBackspacePressed() {
_controller
..text = _controller.text.characters.skipLast(1).toString()
..selection = TextSelection.fromPosition(
TextPosition(offset: _controller.text.length));
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: const Text('表情符号选择器示例应用'),
),
body: Column(
children: [
Expanded(child: Container()),
Container(
height: 66.0,
color: Colors.blue,
child: Row(
children: [
Material(
color: Colors.transparent,
child: IconButton(
onPressed: () {
setState(() {
emojiShowing = !emojiShowing;
});
},
icon: const Icon(
Icons.emoji_emotions,
color: Colors.white,
),
),
),
Expanded(
child: Padding(
padding: const EdgeInsets.symmetric(vertical: 8.0),
child: TextFormField(
controller: _controller,
style: const TextStyle(
fontSize: 20.0, color: Colors.black87),
decoration: InputDecoration(
hintText: '输入消息',
filled: true,
fillColor: Colors.white,
contentPadding: const EdgeInsets.only(
left: 16.0,
bottom: 8.0,
top: 8.0,
right: 16.0),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(50.0),
),
)),
),
),
Material(
color: Colors.transparent,
child: IconButton(
onPressed: () {
// 发送消息
},
icon: const Icon(
Icons.send,
color: Colors.white,
)),
)
],
)),
Offstage(
offstage: !emojiShowing,
child: SizedBox(
height: 250,
child: EmojiPicker(
onEmojiSelected: (Category category, Emoji emoji) {
_onEmojiSelected(emoji);
},
onBackspacePressed: _onBackspacePressed,
config: Config(
columns: 7,
// 修复问题:https://github.com/flutter/flutter/issues/28894
emojiSizeMax: 32 * (Platform.isIOS ? 1.30 : 1.0),
verticalSpacing: 0,
horizontalSpacing: 0,
initCategory: Category.RECENT,
bgColor: const Color(0xFFF2F2F2),
indicatorColor: Colors.blue,
iconColor: Colors.grey,
iconColorSelected: Colors.blue,
progressIndicatorColor: Colors.blue,
backspaceColor: Colors.blue,
skinToneDialogBgColor: Colors.white,
skinToneIndicatorColor: Colors.grey,
enableSkinTones: true,
showRecentsTab: true,
recentsLimit: 28,
noRecentsText: '无最近使用的表情符号',
noRecentsStyle: const TextStyle(
fontSize: 20, color: Colors.black26),
tabIndicatorAnimDuration: kTabScrollDuration,
categoryIcons: const CategoryIcons(),
buttonMode: ButtonMode.MATERIAL)),
),
),
],
),
),
);
}
}
配置选项
gugor_emoji
提供了多种配置选项,以满足不同的需求。以下是一些常用的配置选项及其默认值:
属性 | 描述 | 默认值 |
---|---|---|
columns | 每行的表情符号数量 | 7 |
emojiSizeMax | 表情符号的最大宽度和高度 | 32.0 |
verticalSpacing | 表情符号之间的垂直间距 | 0 |
horizontalSpacing | 表情符号之间的水平间距 | 0 |
initCategory | 初始选中的类别 | Category.RECENT |
bgColor | 控件的背景颜色 | Color(0xFFF2F2F2) |
indicatorColor | 类别指示器的颜色 | Colors.blue |
iconColor | 类别图标颜色 | Colors.grey |
iconColorSelected | 选中时类别图标的颜色 | Colors.blue |
progressIndicatorColor | 加载指示器的颜色 | Colors.blue |
backspaceColor | 返回键按钮的颜色 | Colors.blue |
skinToneDialogBgColor | 皮肤色调对话框的背景颜色 | Colors.white |
skinToneIndicatorColor | 多个皮肤色调表情符号旁边的小三角颜色 | Colors.grey |
enableSkinTones | 启用选择某些表情符号的皮肤色调功能 | true |
showRecentsTab | 显示额外的最近使用表情符号标签 | true |
recentsLimit | 最近使用的表情符号保存限制 | 28 |
noRecentsText | 如果没有最近的表情符号要显示的文本 | “无最近使用的表情符号” |
noRecentsStyle | [noRecentsText] 的文本样式 | TextStyle(fontSize: 20, color: Colors.black26) |
tabIndicatorAnimDuration | 选项卡指示器动画到下一个类别的持续时间 | Duration(milliseconds: 300) |
categoryIcons | 确定每个类别的显示图标。可以通过构造函数设置 | CategoryIcons() |
buttonMode | 选择 Material 或 Cupertino 按钮样式 | ButtonMode.MATERIAL |
返回键
你可以通过添加回调方法 onBackspacePressed: () { }
到 EmojiPicker
小部件来添加返回键。这将使用户更容易删除已添加的表情符号,而无需显示键盘。请参阅示例以了解有关用法的更多详细信息。
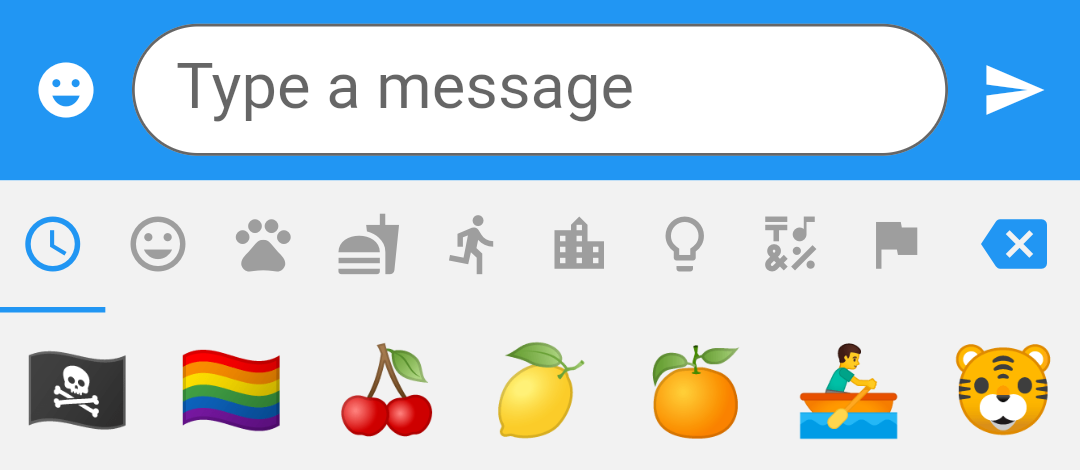
自定义视图
通过设置 customWidget
属性,可以完全自定义视图。如果 Config
中的属性不够,你可以继承自 EmojiPickerBuilder
(推荐但不是必需的)以进行进一步调整。
class CustomView extends EmojiPickerBuilder {
CustomView(Config config, EmojiViewState state) : super(config, state);
@override
_CustomViewState createState() => _CustomViewState();
}
class _CustomViewState extends State<CustomView> {
@override
Widget build(BuildContext context) {
// TODO: 实现构建
// 访问 widget.config 和 widget.state
return Container();
}
}
EmojiPicker(
onEmojiSelected: (Category category, Emoji emoji) { /* ...*/ },
config: Config( /* ...*/ ),
customWidget: (config, state) => CustomView(config, state),
)
使用 EmojiPickerUtils 扩展功能
你可以使用 EmojiPickerUtils
类来扩展 gugor_emoji
的功能。
// 获取最近使用的表情符号
final recentEmojis = await EmojiPickerUtils().getRecentEmojis();
// 根据关键词搜索相关表情符号
final filterEmojiEntities = await EmojiPickerUtils().searchEmoji("face");
// 将表情符号添加到最近使用的列表或增加其计数
final newRecentEmojis = await EmojiPickerUtils().addEmojiToRecentlyUsed(key: key, emoji: emoji);
// 重要:需要相同的 key 实例,类型为 GlobalKey<EmojiPickerState>,以便正常工作
更多关于Flutter表情符号管理插件gugor_emoji的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter表情符号管理插件gugor_emoji的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,下面是一个关于如何在Flutter项目中使用gugor_emoji
插件来管理表情符号的示例代码。gugor_emoji
是一个流行的Flutter插件,用于在应用中集成和管理表情符号。
首先,确保你已经在pubspec.yaml
文件中添加了gugor_emoji
依赖:
dependencies:
flutter:
sdk: flutter
gugor_emoji: ^latest_version # 请替换为实际的最新版本号
然后,运行flutter pub get
来安装依赖。
接下来,你可以在你的Flutter应用中使用gugor_emoji
插件。以下是一个简单的示例,展示如何在应用中显示表情符号选择器并处理选中的表情符号:
import 'package:flutter/material.dart';
import 'package:gugor_emoji/gugor_emoji.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Emoji Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: EmojiScreen(),
);
}
}
class EmojiScreen extends StatefulWidget {
@override
_EmojiScreenState createState() => _EmojiScreenState();
}
class _EmojiScreenState extends State<EmojiScreen> {
String selectedEmoji = '';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Emoji Picker Example'),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'Selected Emoji: $selectedEmoji',
style: TextStyle(fontSize: 24),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
showEmojiPicker(context, onEmojiSelected: (emoji) {
setState(() {
selectedEmoji = emoji;
});
});
},
child: Text('Select Emoji'),
),
],
),
);
}
}
在这个示例中,我们做了以下几件事:
- 添加依赖:在
pubspec.yaml
文件中添加了gugor_emoji
依赖。 - 创建主应用:定义了一个
MyApp
类作为应用的入口。 - 创建主屏幕:定义了一个
EmojiScreen
类,它包含一个文本显示选中的表情符号和一个按钮来打开表情符号选择器。 - 显示选中的表情符号:使用
Text
小部件显示当前选中的表情符号。 - 打开表情符号选择器:使用
showEmojiPicker
函数打开表情符号选择器,并在用户选择表情符号时更新状态。
这个示例展示了如何使用gugor_emoji
插件在Flutter应用中集成表情符号选择器,并处理用户选择的结果。你可以根据需要进一步自定义和扩展这个示例。