Flutter认证授权插件flutter_dauth的使用
Flutter认证授权插件flutter_dauth的使用
特性
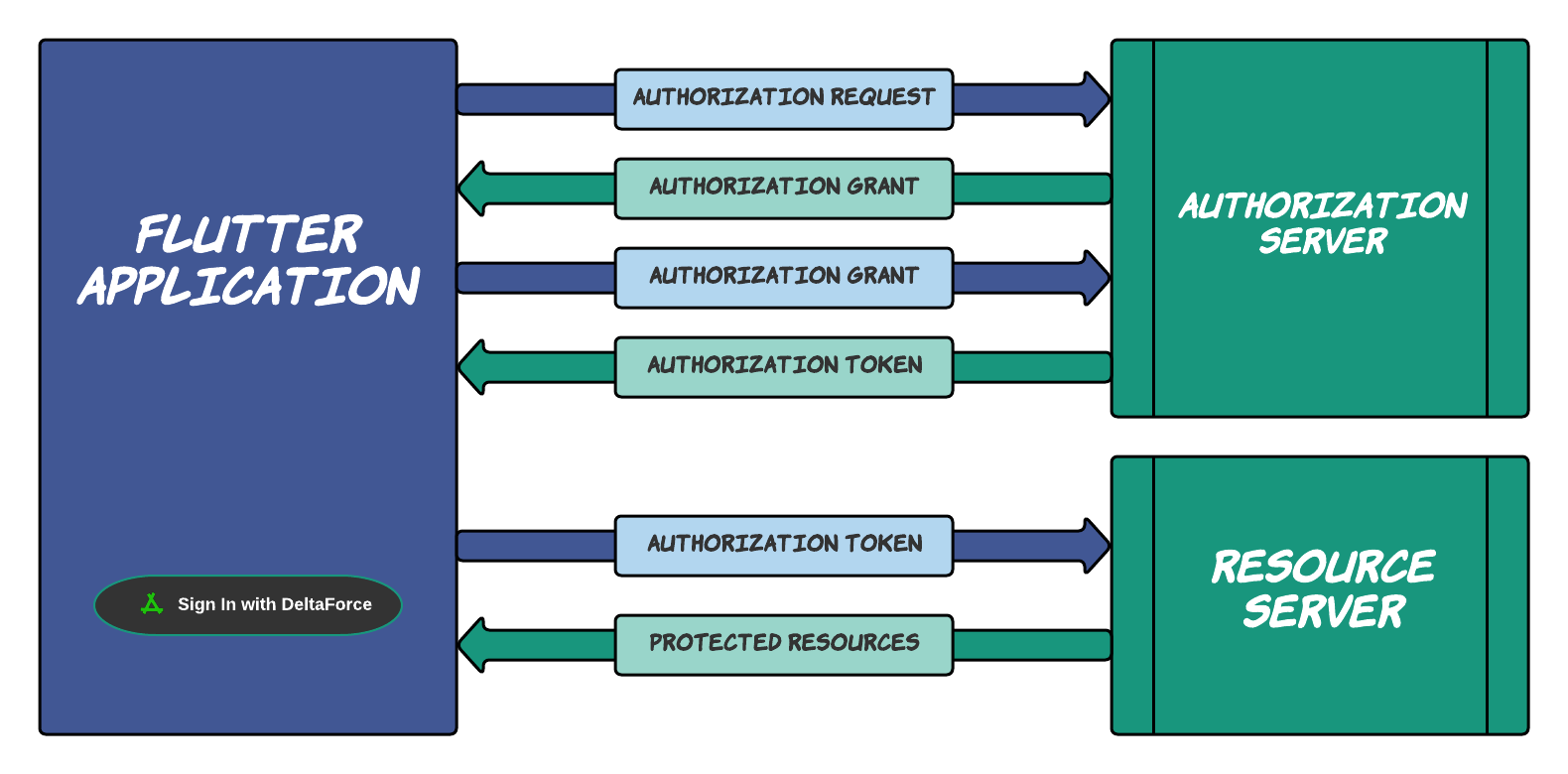
-
此包允许用户通过调用
fetchToken(authorizationRequest)
获取授权令牌,该方法会自动化以下工作流程:- 使用提供的
authorizationRequest
参数生成authorizationUrl
。 - 使用生成的
authorizationUrl
打开一个 WebView 并监听导航请求。 - 允许用户授权客户端应用访问用户的资源。
- 在授权服务器重定向到注册的
redirect_uri
后,通过监听导航请求获取code
。 - 使用
code
作为主体参数自动发起 POST 请求以检索令牌。
- 使用提供的
-
一旦获取了
tokenResponse
,用户可以使用fetchResources(token)
发起 POST 请求,并根据指定的Scope
获取受保护的资源。
数据类型
数据类型 | 参数 | 描述 |
---|---|---|
ResourceResponse | String? tokenType, String? accessToken, String? state, int? expiresIn, String? idToken, String? status, ErrorResponse? errorResponse | 来自 fetchResources() 请求的响应体 |
TokenResponse | String? email, String? id, String? name, String? phoneNumber, String? gender, DateTime? createdAt, DateTime? updatedAt | 来自 fetchToken() 请求的响应体 |
Scope | bool isOpenId, bool isEmail, bool isProfile, bool isUser | 包含四个布尔参数以启用资源访问的范围 |
TokenRequest | String? clientId, String? codeVerifier, String codeChallengeMethod, String? redirectUri, String? responseType, String? grantType, String? state, String? scope, String? nonce | fetchToken() 的请求参数 |
方法
方法 | 参数 |
---|---|
TokenResponse fetchToken() | TokenRequest request |
ResourceResponse fetchResource() | String access_token |
Widget DauthButton() | Function onPressed: (TokenResponse res) {} |
开始使用
要使用此包:
- 在终端运行以下命令
或者flutter pub get flutter_dauth
- 在
pubspec.yaml
文件中添加以下内容dependencies: flutter: sdk: flutter flutter_dauth:
使用示例
以下是一个使用此包的授权码示例。
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
[@override](/user/override)
Widget build(BuildContext context) => const MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
[@override](/user/override)
State<StatefulWidget> createState() => HomeState();
}
class HomeState extends State<HomePage> {
// 用于在 Text() 小部件中作为数据的字符串对象。
String _exampleText = 'Flutter 应用程序';
// 创建一个 TokenRequest 对象
final dauth.TokenRequest _request = TokenRequest(
// 您在注册时由 Dauth 服务器提供的客户端 ID。
clientId: '您的客户端 ID',
// 您在注册时提供给 Dauth 服务器的重定向 URI。
redirectUri: '您的重定向 URI',
// 一个字符串,将与 access_token 一起返回以供客户端侧的令牌验证。
state: 'STATE',
// 设置 isUser 为 true 以从 Dauth 服务器检索用户详细信息。
scope: const dauth.Scope(isUser: true),
// codeChallengeMethod 应根据其需求指定为 `plain` 或 `S256`。
codeChallengeMethod: 'S256');
[@override](/user/override)
Widget build(BuildContext context) => SafeArea(
child: Scaffold(
body: Container(
color: Colors.blue,
child: Stack(
children: [
Center(
child: Text(
_exampleText,
textAlign: TextAlign.center,
style: const TextStyle(fontSize: 20, fontWeight: FontWeight.bold),
)),
Positioned(
left: 50,
right: 50,
bottom: 10,
// DAuth 按钮在按下时返回 TokenResponse 和 ResponseMessage。
child: dauth.DauthButton(
request: _request,
onPressed: (dauth.TokenResponse res) {
// 如果响应成功,则将 exampleText 更改为 Token_TYPE: <YOUR_TOKEN>。
setState(() {
_exampleText = 'Token: ' + res.tokenType.toString();
});
}))
],
),
)));
}
更多关于Flutter认证授权插件flutter_dauth的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter认证授权插件flutter_dauth的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是如何在Flutter应用中使用flutter_dauth
插件来实现认证授权的示例代码。flutter_dauth
是一个用于简化OAuth 2.0和OpenID Connect身份验证流程的Flutter插件。
1. 添加依赖
首先,在你的pubspec.yaml
文件中添加flutter_dauth
依赖:
dependencies:
flutter:
sdk: flutter
flutter_dauth: ^x.y.z # 请替换为最新版本号
然后运行flutter pub get
来安装依赖。
2. 配置OAuth 2.0/OpenID Connect提供者
你需要在你的OAuth 2.0/OpenID Connect提供者那里获取客户端ID、客户端密钥、授权URL、令牌URL等信息。以下是一个配置示例:
import 'package:flutter_dauth/flutter_dauth.dart';
final OAuth2Configuration config = OAuth2Configuration(
clientId: 'your-client-id',
clientSecret: 'your-client-secret', // 注意:有些提供者不需要clientSecret
redirectUri: 'your-redirect-uri',
discoveryDocumentUrl: 'https://your-auth-provider/.well-known/openid-configuration', // 或者直接使用authorizationEndpoint和tokenEndpoint
// authorizationEndpoint: 'https://your-auth-provider/oauth/authorize',
// tokenEndpoint: 'https://your-auth-provider/oauth/token',
scopes: ['openid', 'profile', 'email'],
);
3. 初始化OAuth2服务
接下来,你需要初始化OAuth2服务:
import 'package:flutter_dauth/flutter_dauth.dart';
late OAuth2Service _oauth2Service;
Future<void> initOAuth2Service() async {
_oauth2Service = OAuth2Service(config);
// 加载已经存在的令牌(可选)
final storedCredentials = await _oauth2Service.loadCredentials();
if (storedCredentials != null) {
_oauth2Service.credentials = storedCredentials;
}
}
4. 启动认证流程
启动认证流程通常是通过一个Web视图来完成的:
Future<void> authenticate() async {
try {
final AuthorizationResponse response = await _oauth2Service.authorize();
// 处理响应,比如保存令牌等
await _oauth2Service.saveCredentials(response.credentials);
print('Authenticated successfully: ${response.credentials}');
} catch (e) {
print('Authentication failed: $e');
}
}
5. 使用令牌进行API请求
一旦你获得了令牌,你可以使用它来访问受保护的API资源:
Future<void> fetchUserData() async {
if (_oauth2Service.credentials != null) {
final accessToken = _oauth2Service.credentials!.accessToken;
final response = await http.get(
Uri.parse('https://your-api-endpoint/user-info'),
headers: <String, String>{
'Authorization': 'Bearer $accessToken',
},
);
if (response.statusCode == 200) {
print('User data: ${response.body}');
} else {
print('Failed to fetch user data: ${response.statusCode}');
}
} else {
print('No credentials available.');
}
}
6. 完整示例
下面是一个完整的示例,展示了如何集成flutter_dauth
到你的Flutter应用中:
import 'package:flutter/material.dart';
import 'package:flutter_dauth/flutter_dauth.dart';
import 'package:http/http.dart' as http;
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
late OAuth2Service _oauth2Service;
@override
void initState() {
super.initState();
initOAuth2Service();
}
Future<void> initOAuth2Service() async {
final OAuth2Configuration config = OAuth2Configuration(
clientId: 'your-client-id',
clientSecret: 'your-client-secret',
redirectUri: 'your-redirect-uri',
discoveryDocumentUrl: 'https://your-auth-provider/.well-known/openid-configuration',
scopes: ['openid', 'profile', 'email'],
);
_oauth2Service = OAuth2Service(config);
final storedCredentials = await _oauth2Service.loadCredentials();
if (storedCredentials != null) {
_oauth2Service.credentials = storedCredentials;
}
}
Future<void> authenticate() async {
try {
final AuthorizationResponse response = await _oauth2Service.authorize();
await _oauth2Service.saveCredentials(response.credentials);
print('Authenticated successfully: ${response.credentials}');
} catch (e) {
print('Authentication failed: $e');
}
}
Future<void> fetchUserData() async {
if (_oauth2Service.credentials != null) {
final accessToken = _oauth2Service.credentials!.accessToken;
final response = await http.get(
Uri.parse('https://your-api-endpoint/user-info'),
headers: <String, String>{
'Authorization': 'Bearer $accessToken',
},
);
if (response.statusCode == 200) {
print('User data: ${response.body}');
} else {
print('Failed to fetch user data: ${response.statusCode}');
}
} else {
print('No credentials available.');
}
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter DAuth Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: authenticate,
child: Text('Authenticate'),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: fetchUserData,
child: Text('Fetch User Data'),
),
],
),
),
),
);
}
}
这个示例展示了如何初始化OAuth2服务、启动认证流程以及使用获得的令牌进行API请求。请确保将示例代码中的占位符替换为你自己的OAuth 2.0/OpenID Connect提供者信息。