Flutter自定义绘制或图形处理插件etch的使用
Flutter自定义绘制或图形处理插件etch的使用
✍️ Etch #
一个简化、声明式的CustomPaint实现 #
特性 #
- 以声明方式创建CustomPaint小部件。
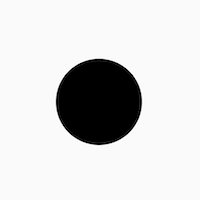
EtchCanvas(
etchElements: [
EtchCircle.alignment(
centerAlignment: Offset.zero,
radius: 50.0,
),
],
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
- 可以使用点或对齐方式来定义点。
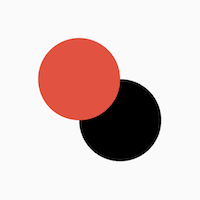
EtchCanvas(
etchElements: [
EtchCircle(
center: Offset(100, 100),
radius: 50.0,
),
EtchCircle.alignment(
centerAlignment: Offset.zero,
radius: 50.0,
etchStyle: EtchStyle(
color: Colors.red,
),
),
],
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
- 支持Paths。
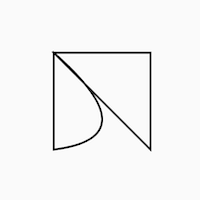
EtchCanvas(
etchElements: [
EtchPath(
etchPathElements: [
EtchPathMoveTo(point: Offset(0, 0)),
EtchPathAddPolygon.alignment(
pointAlignments: [
Offset(-1, -1),
Offset(1, -1),
Offset(1, 1),
],
),
EtchPathQuadraticBezierTo.alignment(
controlPointAlignment: Offset(1, 0.75),
endPointAlignment: Offset(-1, 1),
),
EtchPathClose(),
],
),
],
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
- 轻松处理画布层。
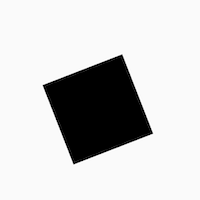
EtchCanvas(
etchElements: [
EtchLayer.rotate(
rotateZ: 1.2,
etchElements: [
EtchRect.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(1, 1),
),
],
),
],
child: SizedBox(
width: 100.0,
height: 100.0,
),
),
- 使用<TweenAnimationBuilder>进行动画。
可以使用<TweenAnimationBuilder>或普通动画控制器轻松添加动画,无需传递进度或其他逻辑。
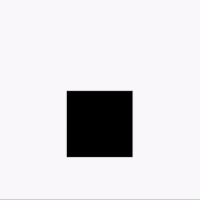
TweenAnimationBuilder<double>(
duration: const Duration(seconds: 1),
tween: Tween(begin: 0, end: 2 * pi),
builder: (context, val, _) {
return EtchCanvas(
etchElements: [
EtchLayer.rotate(
rotateZ: val,
etchElements: [
EtchRect.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(1, 1),
),
],
),
],
child: const SizedBox(
width: 100.0,
height: 100.0,
),
);
}
),
开始使用 #
- 要开始使用,只需在应用中添加
EtchCanvas
。
EtchCanvas(
etchElements: [],
),
所有格式为'Etch----'的元素都可以放入这些元素中(EtchParagraph
,EtchPath
,EtchCircle
等)。
默认构造函数参数通常接受点,而.alignment
构造函数接受对齐方式。对齐方式中,(-1, -1) 是左上角,(1, 1) 是右下角。
EtchCanvas(
etchElements: [
EtchRect.alignment(
topLeftAlignment: Offset.zero,
bottomRightAlignment: Offset(1, 1),
),
EtchOval.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(0, 0),
),
EtchArc.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(1, 1),
startAngle: 0,
sweepAngle: 2,
),
],
),
- 使用
EtchStyle
修改画笔属性。
EtchPath(
etchPathElements: [
//...
],
etchStyle: EtchStyle(
style: PaintingStyle.stroke,
),
),
也可以使用EtchStyle.raw()
提供自己的Paint
对象。
EtchPath(
etchPathElements: [
//...
],
etchStyle: EtchStyle.raw(
Paint()..color = Colors.black..// 添加你的属性,
),
),
- 要向元素中添加路径,使用
EtchPath
。Etch路径元素的命名格式为EtchPath---
(EtchPathAddPolygon
,EtchPathAddArc
,EtchPathCubicTo
等)。
EtchCanvas(
etchElements: [
EtchPath(
etchPathElements: [
EtchPathMoveTo(point: Offset(0, 0)),
EtchPathLine(point: Offset(110, 0)),
EtchPathLine(point: Offset(110, 110)),
EtchPathClose(),
],
etchStyle: EtchStyle(
style: PaintingStyle.stroke,
),
),
],
),
- 要添加图层,使用
EtchLayer
元素。这可以像普通的EtchCanvas
一样包含多个EtchElement。图层可以有独立的变换,不会影响其他部分 - 因此你可以旋转或缩放一层而不影响其他层。EtchLayer
,就像Transform
小部件一样,具有多种内置变换 - 但你也可以使用默认构造函数传递自己的Matrix4
。
EtchCanvas(
etchElements: [
EtchLayer.rotate(
rotateX: 1.2,
etchElements: [
EtchRect.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(1, 1),
),
],
),
EtchLayer.scale(
scale: Offset(1, 2),
etchElements: [
EtchRect.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(1, 1),
),
],
),
EtchLayer(
transform: Matrix4.identity(),
etchElements: [
EtchRect.alignment(
topLeftAlignment: Offset(-1, -1),
bottomRightAlignment: Offset(1, 1),
),
],
),
],
),
额外信息 #
注意:该包目前处于实验阶段,尚未确定完整的指标。如果您在使用过程中遇到任何意外情况,请随时提交问题或PR。
完整示例demo
import 'package:etch/etch.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
// 这个小部件是您的应用的根。
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const DemoPage());
}
}
class DemoPage extends StatefulWidget {
const DemoPage({Key? key}) : super(key: key);
[@override](/user/override)
_DemoPageState createState() => _DemoPageState();
}
class _DemoPageState extends State<DemoPage> {
[@override](/user/override)
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: EtchCanvas(
etchElements: [
EtchLayer.scale(
scale: const Offset(1, 2),
etchElements: [
EtchLine(
start: const Offset(0, 0), end: const Offset(100, 100)),
EtchLine(
start: const Offset(0, 50), end: const Offset(100, 100)),
],
),
EtchPath(
etchPathElements: [
EtchPathMoveTo(point: Offset(0, 0)),
EtchPathLine(point: Offset(110, 0)),
EtchPathLine(point: Offset(110, 110)),
EtchPathClose(),
],
etchStyle: EtchStyle(
style: PaintingStyle.stroke,
),
),
EtchPath(
etchPathElements: [
EtchPathMoveTo(point: const Offset(0, 0)),
EtchPathAddPolygon.alignment(
pointAlignments: [
const Offset(0, 0),
const Offset(1, 0),
const Offset(1, 1),
],
),
EtchPathConicTo.alignment(
controlPointAlignment: const Offset(0.2, 0.4),
endPointAlignment: const Offset(0.5, -0.5),
),
EtchPathClose(),
],
etchStyle: EtchStyle(
style: PaintingStyle.stroke,
),
),
],
child: const SizedBox(
width: 100.0,
height: 100.0,
),
),
),
);
}
}
更多关于Flutter自定义绘制或图形处理插件etch的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
1 回复