Flutter创意进度条插件chaotic_progress的使用
Flutter创意进度条插件chaotic_progress的使用
在本教程中,我们将介绍如何使用Flutter插件chaotic_progress
来创建一个具有独特动画效果的创意进度条。
特性
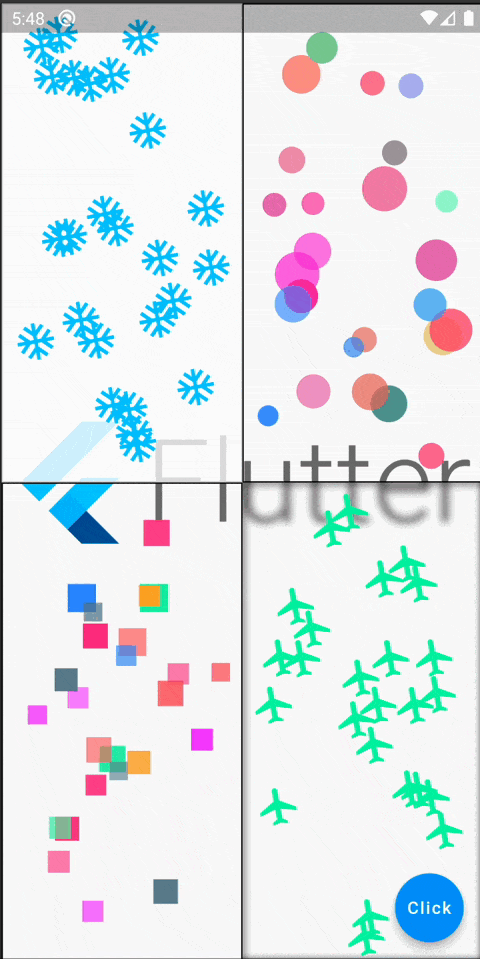
开始使用
在你的Flutter项目的pubspec.yaml
文件中添加以下依赖:
dependencies:
...
chaotic_progress: any
然后,在你的Dart文件中导入该包:
import 'package:chaotic_progress/chaotic_progress.dart';
使用
下面是一个简单的示例代码,展示如何使用ChaoticProgress
插件:
import 'dart:ui';
import 'package:chaotic_progress/chaotic_progress.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const App());
}
class App extends StatelessWidget {
const App({super.key});
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({super.key});
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> with SingleTickerProviderStateMixin {
var _showProgress = true;
late final _animationController = AnimationController(
vsync: this,
duration: const Duration(seconds: 5),
)..repeat();
@override
Widget build(BuildContext context) {
return Scaffold(
body: Stack(
alignment: Alignment.center,
children: [
const FlutterLogo(
size: 300,
style: FlutterLogoStyle.horizontal,
),
ChaoticProgress(
visible: _showProgress,
// backgroundColor: Colors.white.withOpacity(0.5),
filter: ImageFilter.blur(sigmaX: 3.0, sigmaY: 3.0),
alignCurve: Curves.linear,
duration: const Duration(seconds: 4),
shapeBuilder: (context, index, color, scale) => AnimatedBuilder(
animation: _animationController,
builder: (_, child) => Transform.rotate(
angle: -2 * 3.1415926 * _animationController.value,
child: child,
),
child: AnimatedScale(
scale: scale,
duration: const Duration(seconds: 1),
child: const Icon(
Icons.ac_unit,
color: Colors.lightBlueAccent,
size: 36,
),
),
),
),
],
),
floatingActionButton: FloatingActionButton(
onPressed: () {
setState(() {
_showProgress = !_showProgress;
_showProgress
? _animationController.repeat()
: _animationController.stop(canceled: false);
});
},
child: const Text('Click'),
),
);
}
@override
void dispose() {
_animationController.dispose();
super.dispose();
}
}
参数说明
以下是ChaoticProgress
类的所有属性及其说明:
class ChaoticProgress extends StatefulWidget {
const ChaoticProgress({
super.key,
this.numberOfShapes = 75,
this.visible = true,
this.minShapeSize = 15.0,
this.maxShapeSize = 40.0,
this.minShapeOpacity = 0.5,
this.maxShapeOpacity = 1.0,
this.colors = const [
Colors.redAccent,
Colors.greenAccent,
Colors.blueAccent,
],
this.shape = BoxShape.circle,
this.alignCurve = Curves.easeInBack,
this.figureCurve = Curves.linearToEaseOut,
this.duration = const Duration(milliseconds: 1500),
this.filter,
this.backgroundColor,
this.shapeBuilder,
});
/// 显示的形状数量。
final int numberOfShapes;
/// 控制是否显示形状。
final bool visible;
/// 形状的最小大小。
final double minShapeSize;
/// 形状的最大大小。
final double maxShapeSize;
/// 形状透明度的最小值。
///
/// 透明度为1.0表示完全不透明。透明度为0.0表示完全透明(即不可见)。
final double minShapeOpacity;
/// 形状透明度的最大值。
///
/// 透明度为1.0表示完全不透明。透明度为0.0表示完全透明(即不可见)。
final double maxShapeOpacity;
/// 形状的形状。
final BoxShape shape;
/// 随机选择显示形状的颜色列表。
final List<Color> colors;
/// 当动画参数时应用的曲线。
final Curve figureCurve;
/// 当动画对齐参数时应用的曲线。
final Curve alignCurve;
/// 在绘制子元素之前应用于现有绘制内容的图像滤镜。
///
/// 例如,可以使用[ImageFilter.blur]创建背景模糊效果。
final ImageFilter? filter;
/// 动画形状的对齐和大小持续时间。
final Duration duration;
/// 背景颜色。
///
/// [backgroundColor]在形状下方绘制。
final Color? backgroundColor;
/// 可以返回自定义形状小部件的构建器。
final ChaoticProgressShapeBuilder? shapeBuilder;
}
更多关于Flutter创意进度条插件chaotic_progress的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter创意进度条插件chaotic_progress的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,下面是一个关于如何使用Flutter中的chaotic_progress
插件来创建创意进度条的代码示例。这个插件提供了多种风格的进度条,适合为应用增加一些视觉上的趣味性。
首先,你需要在pubspec.yaml
文件中添加chaotic_progress
依赖:
dependencies:
flutter:
sdk: flutter
chaotic_progress: ^最新版本号 # 请替换为实际最新版本号
然后运行flutter pub get
来安装依赖。
接下来,你可以在你的Dart文件中使用chaotic_progress
提供的各种进度条组件。以下是一个简单的示例,展示如何使用其中的一些进度条:
import 'package:flutter/material.dart';
import 'package:chaotic_progress/chaotic_progress.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Chaotic Progress Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: Scaffold(
appBar: AppBar(
title: Text('Chaotic Progress Demo'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
SizedBox(height: 20),
ChaoticProgress(
progress: 0.5, // 进度值,0到1之间
type: ChaoticProgressType.cubeGrid, // 进度条类型
color: Colors.blue, // 进度条颜色
),
SizedBox(height: 20),
ChaoticProgress(
progress: 0.75,
type: ChaoticProgressType.lineScaleParty,
color: Colors.green,
),
SizedBox(height: 20),
ChaoticProgress(
progress: 0.3,
type: ChaoticProgressType.circle,
color: Colors.red,
),
SizedBox(height: 20),
ChaoticProgress(
progress: 0.9,
type: ChaoticProgressType.fadingCircle,
color: Colors.purple,
),
],
),
),
),
);
}
}
在这个示例中,我们创建了一个简单的Flutter应用,其中包含了四种不同类型的创意进度条:
cubeGrid
:立方体网格动画。lineScaleParty
:线条缩放派对动画。circle
:圆形进度条。fadingCircle
:淡入淡出圆形动画。
你可以通过修改progress
属性来控制进度条的进度,通过type
属性来选择不同的动画效果,以及通过color
属性来设置进度条的颜色。
chaotic_progress
插件提供了多种进度条类型,你可以在chaotic_progress的官方文档(请替换为实际链接)中找到完整的类型列表和更多使用示例。
希望这个示例能帮助你快速上手chaotic_progress
插件的使用!