Flutter界面快照插件simple_widget_snapshot的使用
Flutter界面快照插件simple_widget_snapshot的使用
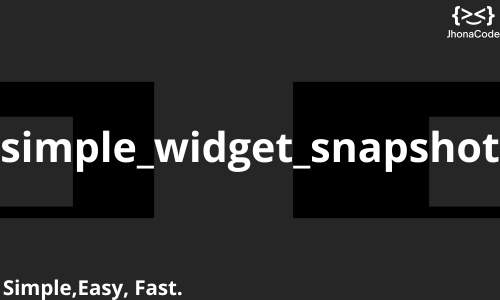
功能
通过我们的库轻松地捕获小部件的快照并将其转换为图像或PDF文件。我们的库提供了高级自定义选项、直观的API,并与流行的Flutter包无缝集成,使您可以完全控制快照生成过程。
安装
要安装simple_widget_snapshot
,在你的pubspec.yaml
文件中添加以下依赖:
dependencies:
simple_widget_snapshot: ^2.0.0
运行以下命令来获取依赖项:
flutter pub add simple_widget_snapshot
使用
WidgetSnapshot 使用指南
WidgetSnapshot
类提供了一种简单的方法来捕获一个作为图像的小部件。
本指南将演示如何在您的Flutter应用程序中使用它。
基本用法
- 创建一个
GlobalKey
以标识您想要捕获的小部件:
final GlobalKey _globalKey = GlobalKey();
- 将您想要捕获的小部件包裹在一个
RepaintBoundary
中,并分配GlobalKey
:
RepaintBoundary(
key: _globalKey,
child: YourWidget(),
)
- 调用
WidgetSnapshot.capture()
方法来捕获小部件:
WidgetSnapshot.capture(_globalKey, pixelRatio: 3.0).then((result) {
// 使用捕获的图像数据
setState(() {
_byteData = result.byteData;
});
});
示例
以下是在Flutter应用中使用WidgetSnapshot
的一个完整示例:
class _MyHomePageState extends State<MyHomePage> {
ByteData? _byteData;
[@override](/user/override)
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('WidgetSnapshot Demo')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
RepaintBoundary(
key: _globalKey,
child: Container(
height: 200,
width: 200,
color: Colors.blue,
child: Center(child: Text('Capture me!')),
),
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
WidgetSnapshot.capture(_globalKey, pixelRatio: 3.0).then((result) {
setState(() {
_byteData = result.byteData;
});
});
},
child: Text('Capture Widget'),
),
SizedBox(height: 20),
if (_byteData != null)
Container(
height: 200,
width: 200,
child: Image.memory(_byteData!.buffer.asUint8List()),
),
],
),
),
);
}
}
更多关于Flutter界面快照插件simple_widget_snapshot的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter界面快照插件simple_widget_snapshot的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是如何在Flutter项目中使用simple_widget_snapshot
插件来捕获界面快照的详细步骤和代码示例。
1. 添加依赖
首先,你需要在你的pubspec.yaml
文件中添加simple_widget_snapshot
依赖:
dependencies:
flutter:
sdk: flutter
simple_widget_snapshot: ^最新版本号 # 请替换为实际发布的最新版本号
然后运行flutter pub get
来安装依赖。
2. 导入插件
在你需要使用快照功能的Dart文件中导入simple_widget_snapshot
:
import 'package:simple_widget_snapshot/simple_widget_snapshot.dart';
3. 使用插件
以下是一个完整的示例,展示如何捕获一个Widget
的快照并将其保存为PNG文件:
import 'package:flutter/material.dart';
import 'package:simple_widget_snapshot/simple_widget_snapshot.dart';
import 'dart:ui' as ui;
import 'dart:io';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Simple Widget Snapshot Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Tap the button to capture snapshot'),
SizedBox(height: 20),
ElevatedButton(
onPressed: () async {
// 要捕获的Widget
Widget widgetToCapture = Column(
children: <Widget>[
Text('Hello, Flutter!'),
Text('This is a snapshot example.'),
],
);
// 捕获快照
ui.Image? snapshotImage = await SimpleWidgetSnapshot.captureWidget(
widget: widgetToCapture,
backgroundColor: Colors.white, // 可选,设置背景颜色
);
if (snapshotImage != null) {
// 将快照保存为PNG文件
final byteData = await snapshotImage.toByteData(
format: ui.ImageByteFormat.png,
);
final Uint8List pngBytes = byteData!.buffer.asUint8List();
// 指定文件路径
final File file = File('/path/to/save/snapshot.png');
// 写入文件
await file.writeAsBytes(pngBytes);
// 打印成功消息
print('Snapshot saved to ${file.path}');
} else {
print('Failed to capture snapshot');
}
},
child: Text('Capture Snapshot'),
),
],
),
),
),
);
}
}
注意事项
- 文件路径:在示例中,
/path/to/save/snapshot.png
需要替换为你实际希望保存快照的路径。确保你的应用有权限写入该路径。 - UI线程:
SimpleWidgetSnapshot.captureWidget
是一个耗时操作,因此建议在异步函数中使用,以避免阻塞UI线程。 - 背景颜色:
backgroundColor
参数是可选的,你可以根据需要设置背景颜色。
通过以上步骤,你应该能够在Flutter应用中成功捕获Widget的快照并将其保存为PNG文件。