Flutter轮播页面插件carousel_page_view的使用
Flutter轮播页面插件carousel_page_view的使用
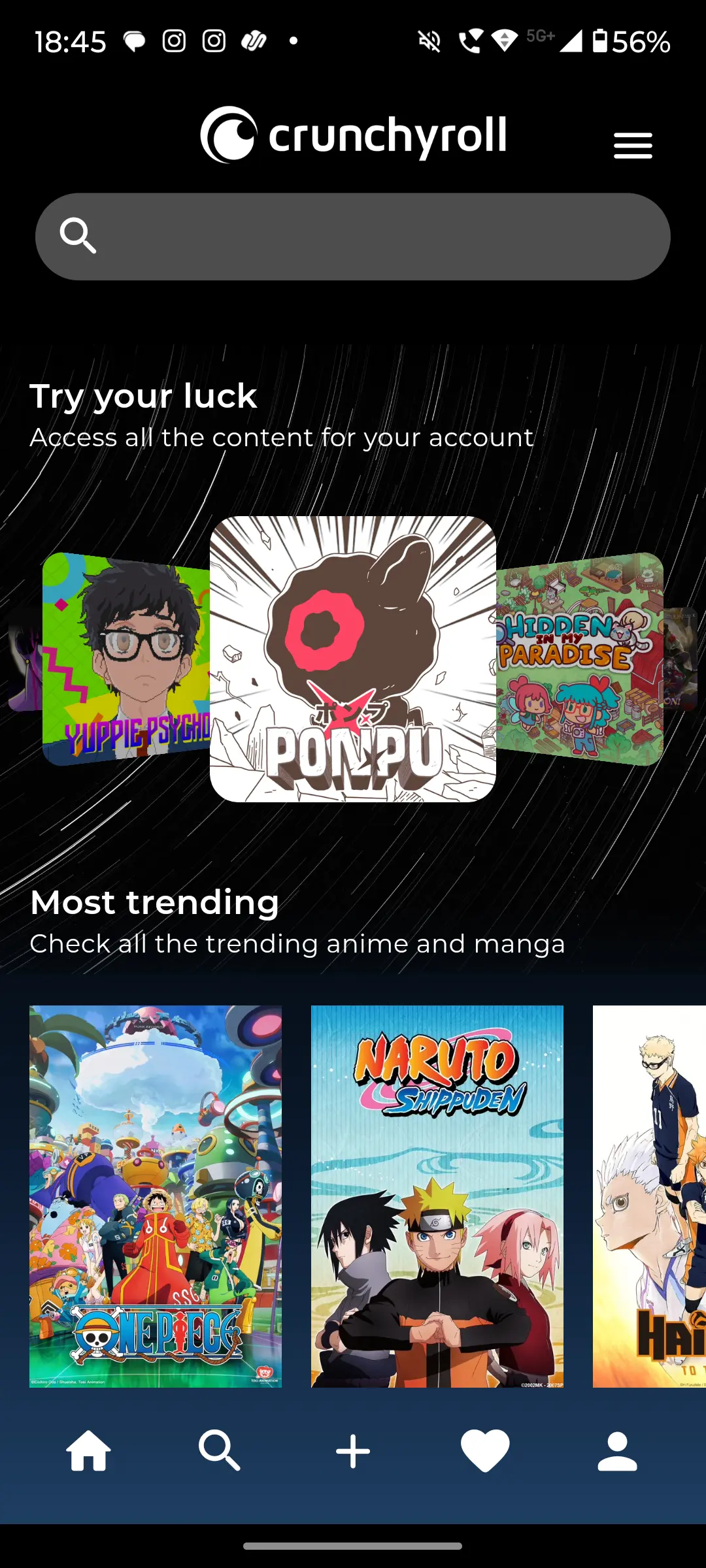
特性
创建具有视图页的3D风格轮播。该组件在Z轴上移动并在X轴上旋转,赋予其3D效果。
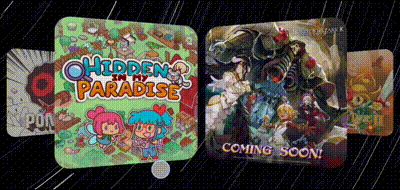
开始使用
在你的 pubspec.yaml
文件中添加依赖:
dependencies:
carousel_page_view: ^1.0.2
在你的 Dart 文件中导入包:
import 'package:carousel_page_view/carousel_plus.dart';
使用方法
将 CarouselPlus
添加到你的小部件中,并传递你想要显示的小部件列表。
import 'package:carousal_test/generated/assets.dart';
import 'package:carousel_page_view/carousel_plus.dart';
import 'package:flutter/material.dart';
const carouselImages = [
Assets.sqImages1,
Assets.sqImages2,
Assets.sqImages3,
Assets.sqImages4,
];
class HomeScreen extends StatelessWidget {
const HomeScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black,
body: Center(
child: SizedBox(
width: MediaQuery.of(context).size.width,
height: 180,
child: CarouselPlus(
childSize: 100,
onCenterItemPressed: (index) {
print('Center item pressed: $index');
},
children: List.generate(carouselImages.length, (index) {
return Image.asset(
carouselImages[index],
fit: BoxFit.cover,
);
}),
),
),
),
);
}
}
在这个示例中,我们创建了一个包含四张图片的轮播组件。CarouselPlus
接收一个 children
列表,其中每个元素都是一个小部件(在这个例子中是 Image
)。childSize
参数用于设置每个轮播项的大小。onCenterItemPressed
回调函数会在当前中心项被点击时触发,并打印出相应的索引。
未来路线图
- 添加更多配置选项,如旋转、缩放等。
更多关于Flutter轮播页面插件carousel_page_view的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter轮播页面插件carousel_page_view的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,下面是一个关于如何使用Flutter轮播页面插件carousel_page_view
的示例代码。这个示例将展示如何在一个Flutter应用中集成和使用carousel_page_view
来实现图片轮播效果。
首先,确保你的pubspec.yaml
文件中已经添加了carousel_page_view
依赖:
dependencies:
flutter:
sdk: flutter
carousel_page_view: ^0.1.0 # 请确保使用最新版本号
然后,运行flutter pub get
来安装依赖。
接下来是主要的Dart代码,展示如何使用carousel_page_view
:
import 'package:flutter/material.dart';
import 'package:carousel_page_view/carousel_page_view.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Carousel Page View Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: CarouselDemoPage(),
);
}
}
class CarouselDemoPage extends StatelessWidget {
final List<String> imageUrls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg',
// 添加更多图片URL
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Carousel Page View Demo'),
),
body: Center(
child: CarouselPageView(
items: imageUrls.map((url) {
return CarouselPageViewItemBuilder(
builder: (context) {
return Container(
decoration: BoxDecoration(
image: DecorationImage(
image: NetworkImage(url),
fit: BoxFit.cover,
),
),
);
},
);
}).toList(),
autoPlay: true, // 自动播放
autoPlayInterval: Duration(seconds: 3), // 自动播放间隔
autoPlayDirection: CarouselAutoPlayDirection.forward, // 播放方向
indicatorType: CarouselIndicatorType.dot, // 指示器类型
dotIndicatorConfig: DotIndicatorConfig(
dotActiveColor: Colors.red,
dotSize: 10.0,
dotSpacing: 10.0,
dotDecorator: (dotIndex, isActive) {
return Container(
decoration: BoxDecoration(
shape: BoxShape.circle,
color: isActive ? Colors.red : Colors.grey.withOpacity(0.5),
),
width: isActive ? 14.0 : 10.0,
height: isActive ? 14.0 : 10.0,
);
},
),
),
),
);
}
}
在这个示例中,我们创建了一个简单的Flutter应用,其中包含一个使用carousel_page_view
实现的图片轮播页面。以下是代码的关键点:
- 依赖引入:在
pubspec.yaml
中添加了carousel_page_view
依赖。 - 数据准备:在
CarouselDemoPage
类中准备了一个图片URL列表。 - 构建轮播页面:使用
CarouselPageView
组件,并通过CarouselPageViewItemBuilder
将每个图片URL映射为一个页面项。 - 配置轮播属性:设置了自动播放、播放间隔、播放方向以及指示器类型等配置。
你可以根据需要调整这些配置,例如改变自动播放间隔、指示器样式等,以满足你的具体需求。希望这个示例对你有所帮助!