Flutter文档选择插件document_picker的使用
Flutter文档选择插件document_picker的使用
document_picker 0.0.6
document_picker
是一个文档选择小部件,带有最新功能,可以在您的项目设计中使用。
目的
本项目的目的是提供一个终极小部件来选择/捕获图像。
通常,您需要花费几乎一整天的时间来编写用于电子身份验证(e-KYC)或证明(拍摄的照片、从图库中选择的图像或签名)以上传的代码。
这里我尝试通过提供一个可以导入并按您所需使用的包来节省您的时间。
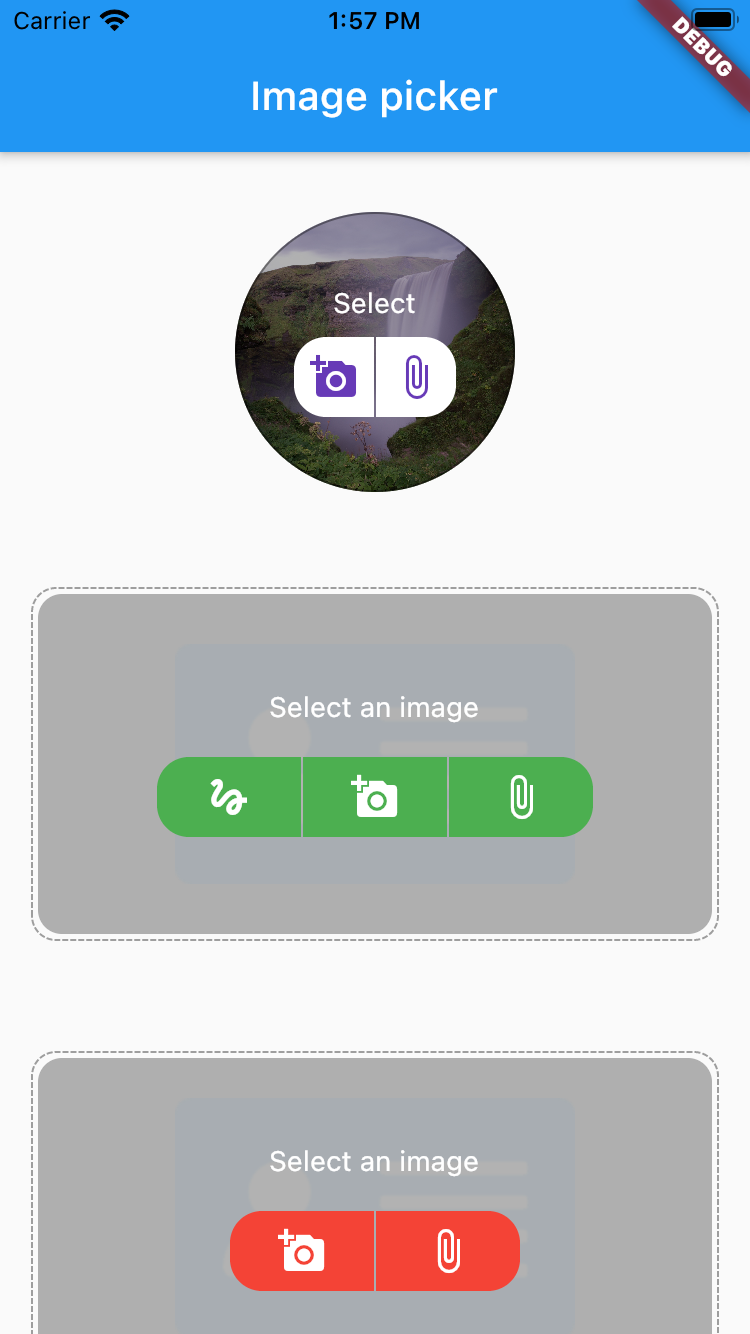
您需要遵循以下步骤:
对于iOS
打开您的 ios/Runner/info.plist
以添加相机和图库权限:
<key>NSCameraUsageDescription</key>
<string>为了进行电子身份验证和证明而拍摄文档照片</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>为了进行电子身份验证和证明而选择现有的文档照片</string>
对于Android
更新 build.gradle
中的最小SDK版本为21:
minSdkVersion 21
打开您的 android/app/src/main/AndroidManifest.xml
以添加相机、图库和裁剪器的权限和活动:
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
<activity
android:name="com.yalantis.ucrop.UCropActivity"
android:screenOrientation="portrait"
android:theme="@style/Theme.AppCompat.Light.NoActionBar"/>
安装
在您的包的 pubspec.yaml
文件中添加以下依赖项:
dependencies:
document_picker: ^0.0.6
示例用法
import 'package:document_picker/document_picker.dart';
// 在你的代码中使用 ProfilePicture 和 DocumentSelector 小部件
ProfilePicture(
url: '',
editable: true,
onFileSelection: (file) {},
),
SizedBox(height: 40),
DocumentSelector(
url: '',
editable: true,
onFileSelection: (File? file) {
print(file);
},
onErrorMessage: (String? message) {
print(message);
},
),
完整示例代码
import 'dart:io';
import 'package:document_picker/document_picker.dart';
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// 这个小部件是您应用的根组件。
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(title: '文档选择器'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, required this.title}) : super(key: key);
final String title;
[@override](/user/override)
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
[@override](/user/override)
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: ListView(
children: [
SizedBox(height: 30),
ProfilePicture(
url: '',
editable: true,
onFileSelection: (file) {},
onErrorMessage: (str) {
print(str);
},
),
SizedBox(height: 40),
DocumentSelector(
url: '',
editable: true,
onFileSelection: (File? file) {
print(file);
},
onErrorMessage: (String? message) {
print(message);
},
),
SizedBox(height: 40),
DocumentSelector(
url: '',
editable: true,
height: 90,
iconsBackgroundColor: Colors.red,
handwritingVisible: false,
onFileSelection: (File? file) {
print(file);
},
onErrorMessage: (message) {
print(message);
},
),
SizedBox(height: 100),
],
),
),
);
}
}
更多关于Flutter文档选择插件document_picker的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter文档选择插件document_picker的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是一个关于如何在Flutter应用中使用document_picker
插件来选择文档的示例代码。这个插件允许用户从设备的文件系统中选择一个文档。
首先,你需要在你的pubspec.yaml
文件中添加document_picker
依赖:
dependencies:
flutter:
sdk: flutter
document_picker: ^2.0.0 # 请检查最新版本号
然后,运行flutter pub get
来安装依赖。
接下来,在你的Flutter应用中,你可以按照以下步骤来使用document_picker
插件:
- 导入必要的包:
import 'package:flutter/material.dart';
import 'package:document_picker/document_picker.dart';
- 创建一个按钮来触发文档选择:
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: DocumentPickerScreen(),
);
}
}
class DocumentPickerScreen extends StatefulWidget {
@override
_DocumentPickerScreenState createState() => _DocumentPickerScreenState();
}
class _DocumentPickerScreenState extends State<DocumentPickerScreen> {
String? selectedFilePath;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Document Picker Example'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
ElevatedButton(
onPressed: _pickDocument,
child: Text('Pick Document'),
),
if (selectedFilePath != null)
Text(
'Selected Document Path: $selectedFilePath',
style: TextStyle(fontSize: 18),
),
],
),
),
);
}
Future<void> _pickDocument() async {
final result = await DocumentPicker.platform.pickFiles(
allowMultiple: false,
type: DocumentType.any,
);
if (result != null) {
if (result.files.isNotEmpty) {
FilePickerFile file = result.files.first;
setState(() {
selectedFilePath = file.path;
});
}
}
}
}
在这个示例中,我们创建了一个简单的Flutter应用,其中包含一个按钮。当用户点击按钮时,会调用_pickDocument
函数来触发文档选择对话框。选择的文档路径会显示在按钮下方。
注意事项:
-
权限处理:在Android和iOS上,你需要处理文件访问权限。对于Android,你可能需要在
AndroidManifest.xml
中添加相应的权限声明。对于iOS,你可能需要在Info.plist
中添加相应的权限说明。document_picker
插件通常会自动处理这些权限请求,但你应该确保你的应用符合相应的平台要求。 -
错误处理:在实际应用中,你应该添加错误处理逻辑来处理用户取消选择或选择文件时出现的错误。
-
插件版本:请确保你使用的是最新版本的
document_picker
插件,因为插件的API可能会随着版本的更新而变化。
希望这个示例代码能帮助你在Flutter应用中使用document_picker
插件!