Flutter进度对话框插件progress_dialog2的使用
Flutter进度对话框插件progress_dialog2的使用
progress_dialog2
感谢您使用本插件。由于一些原因,该插件已停止维护,请找到其他库来完成本插件的功能。我相信这些库会更有帮助。您可以在此处查看仓库链接:OTS (Over the screen)
支持的Dart版本
Dart SDK 版本 >= 2.19.2
演示
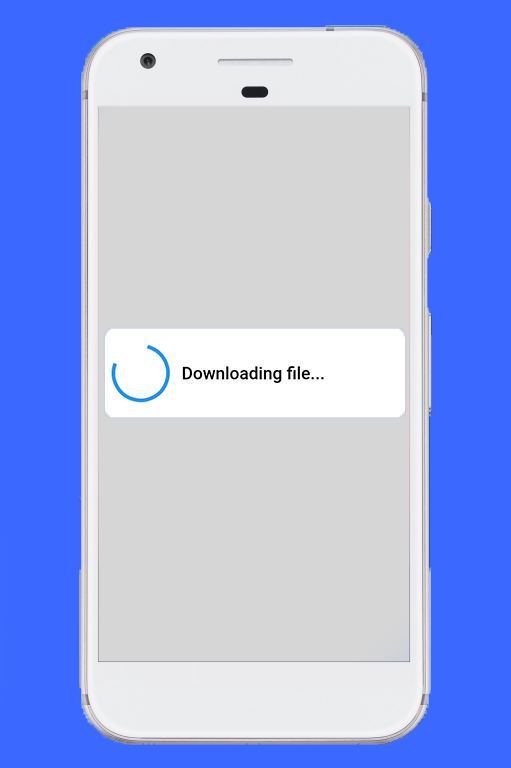
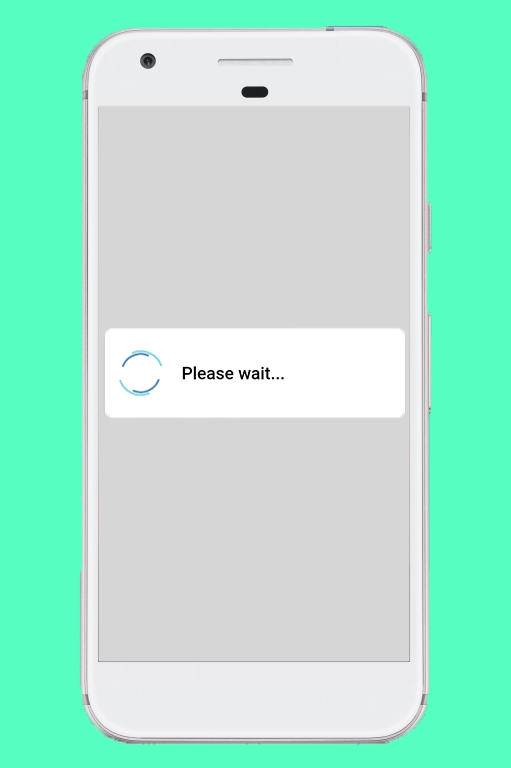
安装
在 pubspec.yaml
文件中添加以下依赖:
dependencies:
progress_dialog2: ^1.0.0
如何使用
在您的 Dart 文件中导入插件:
import 'package:progress_dialog2/progress_dialog2.dart';
创建并初始化一个 ProgressDialog
对象,并将上下文传递给它:
- 初始化
ProgressDialog
对象
final ProgressDialog pr = ProgressDialog(context);
- 默认情况下,它是一个简单的对话框,用于显示一些消息。如果您想用它来显示进度百分比,可以指定可选的
type
参数,并指定是否希望对话框在按下返回键时可取消(可选)。
// 对于普通对话框
pr = ProgressDialog(context, type: ProgressDialogType.Normal, isDismissible: true/false, showLogs: true/false);
// 对于显示进度百分比
pr = ProgressDialog(context, type: ProgressDialogType.Download, isDismissible: true/false, showLogs: true/false);
注意:请在有上下文可用的地方初始化
ProgressDialog
。
- 样式化进度对话框(可选)
pr.style(
message: 'Downloading file...', // 消息文本
borderRadius: 10.0, // 圆角半径
backgroundColor: Colors.white, // 背景颜色
progressWidget: CircularProgressIndicator(), // 进度条小部件
elevation: 10.0, // 阴影高度
insetAnimCurve: Curves.easeInOut, // 内边距动画曲线
progress: 0.0, // 当前进度
textDirection: TextDirection.rtl, // 文字方向
maxProgress: 100.0, // 最大进度
progressTextStyle: TextStyle(
color: Colors.black, fontSize: 13.0, fontWeight: FontWeight.w400), // 进度文本样式
messageTextStyle: TextStyle(
color: Colors.black, fontSize: 19.0, fontWeight: FontWeight.w600) // 消息文本样式
);
注意:您不需要使用所有参数,它们都是可选的。
- 显示进度对话框
await pr.show();
- 动态更新显示的内容
pr.update(
progress: 50.0, // 更新进度
message: "Please wait...", // 更新消息
progressWidget: Container(
padding: EdgeInsets.all(8.0),
child: CircularProgressIndicator()), // 更新进度条小部件
maxProgress: 100.0, // 最大进度
progressTextStyle: TextStyle(
color: Colors.black, fontSize: 13.0, fontWeight: FontWeight.w400), // 进度文本样式
messageTextStyle: TextStyle(
color: Colors.black, fontSize: 19.0, fontWeight: FontWeight.w600) // 消息文本样式
);
注意:您不需要使用所有参数,它们都是可选的。
- 隐藏进度对话框
pr.hide().then((isHidden) {
print(isHidden);
});
// 或者
await pr.hide();
注意:导航到下一个屏幕必须在
Future
-hide()
完成之后进行。查看这里 的示例。
检查进度对话框是否正在显示
bool isProgressDialogShowing = pr.isShowing();
print(isProgressDialogShowing);
使用自定义主体
pr = ProgressDialog(
context,
type: ProgressDialogType.Normal,
isDismissible: true,
/// 自定义主体
customBody: LinearProgressIndicator(
valueColor: AlwaysStoppedAnimation<Color>(Colors.blueAccent),
backgroundColor: Colors.white,
),
);
更多关于Flutter进度对话框插件progress_dialog2的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter进度对话框插件progress_dialog2的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是如何在Flutter项目中使用progress_dialog2
插件来显示进度对话框的一个示例代码案例。
首先,你需要在你的pubspec.yaml
文件中添加progress_dialog2
依赖:
dependencies:
flutter:
sdk: flutter
progress_dialog2: ^4.0.0 # 请检查最新版本号
然后运行flutter pub get
来安装依赖。
接下来,你可以在你的Flutter项目中创建一个进度对话框。以下是一个完整的示例,展示如何在点击按钮时显示进度对话框,并在模拟的异步操作完成后关闭对话框。
import 'package:flutter/material.dart';
import 'package:progress_dialog2/progress_dialog2.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Progress Dialog Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
late ProgressDialog progressDialog;
@override
void initState() {
super.initState();
progressDialog = ProgressDialog(context,
type: ProgressDialogType.Normal,
isDismissible: false,
showLogs: true,
);
}
Future<void> _showProgressDialog() async {
await progressDialog.show(
title: 'Loading',
message: 'Please wait...',
barColor: Colors.blue,
);
// Simulate a long-running task
await Future.delayed(Duration(seconds: 3));
// Once the task is complete, dismiss the dialog
progressDialog.hide();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Progress Dialog Example'),
),
body: Center(
child: ElevatedButton(
onPressed: _showProgressDialog,
child: Text('Show Progress Dialog'),
),
),
);
}
}
在这个示例中:
- 我们首先在
pubspec.yaml
文件中添加了progress_dialog2
依赖。 - 在
MyApp
类中,我们定义了应用程序的入口。 - 在
MyHomePage
类中,我们创建了一个进度对话框实例progressDialog
,并在initState
方法中初始化它。 - 我们定义了一个
_showProgressDialog
方法,该方法显示进度对话框,模拟一个耗时操作(使用Future.delayed
),然后在操作完成后隐藏对话框。 - 在
build
方法中,我们创建了一个按钮,当点击该按钮时,调用_showProgressDialog
方法来显示进度对话框。
这样,你就可以在你的Flutter应用程序中使用progress_dialog2
插件来显示进度对话框了。