Flutter聊天界面插件chatview2的使用
Flutter聊天界面插件chatview2的使用
概览
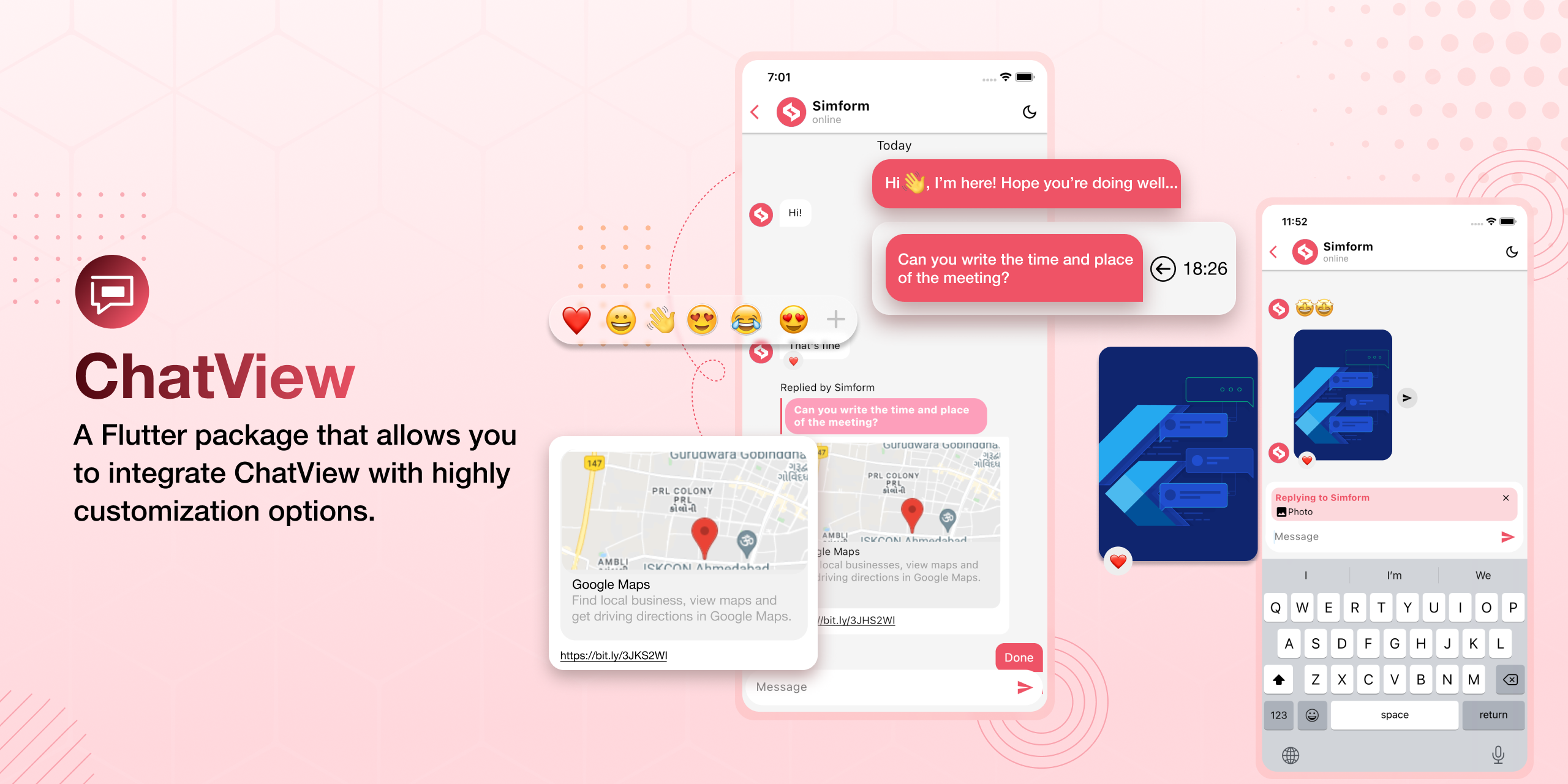
chatview2
是一个Flutter包,允许你集成具有高度定制选项的聊天视图,如一对一聊天、群组聊天、消息反应、回复消息、链接预览以及整体视图的配置。
对于网页演示,请访问 Chat View Example。
预览
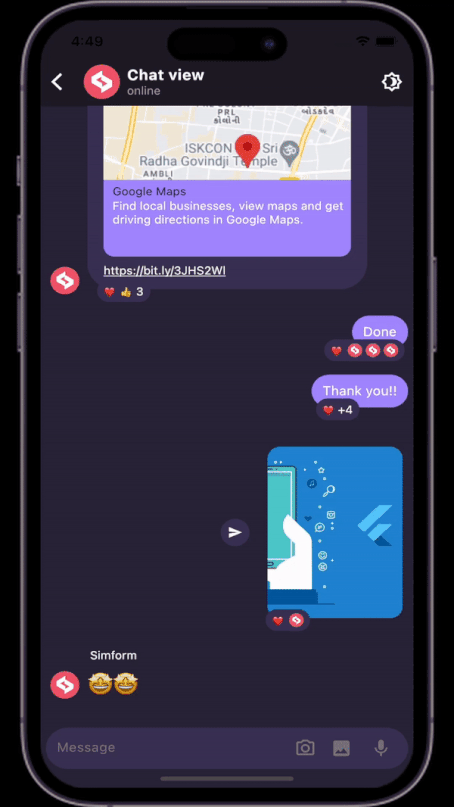
安装
- 在
pubspec.yaml
文件中添加依赖项:
dependencies:
chatview2: <latest-version>
- 获取最新版本,请查看
pub.dev
上的 ‘Installing’ 标签。
- 导入包:
import 'package:chatview2/chatview2.dart';
- 添加一个聊天控制器:
final chatController = ChatController(
initialMessageList: messageList,
scrollController: ScrollController(),
chatUsers: [ChatUser(id: '2', name: 'Simform')],
);
- 添加
ChatView
组件:
ChatView(
currentUser: ChatUser(id: '1', name: 'Flutter'),
chatController: chatController,
onSendTap: onSendTap,
chatViewState: ChatViewState.hasMessages, // 添加此状态以确保数据可用。
)
- 添加消息列表:
List<Message> messageList = [
Message(
id: '1',
message: "Hi",
createdAt: createdAt,
sendBy: userId,
),
Message(
id: '2',
message: "Hello",
createdAt: createdAt,
sendBy: userId,
),
];
- 添加发送消息回调:
void onSendTap(String message, ReplyMessage replyMessage, MessageType messageType) {
final message = Message(
id: '3',
message: "How are you",
createdAt: DateTime.now(),
sendBy: currentUser.id,
replyMessage: replyMessage,
messageType: messageType,
);
chatController.addMessage(message);
}
注意:你可以通过 messageType
参数评估消息类型,并根据该类型执行操作。
消息类型兼容性
消息类型 | Android | iOS | MacOS | Web | Linux | Windows |
---|---|---|---|---|---|---|
文本消息 | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ |
图片消息 | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ |
语音消息 | ✔️ | ✔️ | ❌ | ❌ | ❌ | ❌ |
自定义消息 | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ | ✔️ |
平台特定配置
对于图片选择器
iOS
在 <project root>/ios/Runner/Info.plist
文件中添加以下键:
<key>NSCameraUsageDescription</key>
<string>用于演示图片选择器插件</string>
<key>NSMicrophoneUsageDescription</key>
<string>用于捕获音频以供图片选择器插件使用</string>
<key>NSPhotoLibraryUsageDescription</key>
<string>用于演示图片选择器插件</string>
对于语音消息
iOS
在 ios/Runner/Info.plist
文件中添加以下行:
<key>NSMicrophoneUsageDescription</key>
<string>此应用需要麦克风权限。</string>
在 Podfile
中添加以下行:
platform :ios, '10.0'
Android
在 android/app/build.gradle
文件中将最小Android SDK版本更改为21(或更高):
minSdkVersion 21
在 AndroidManifest.xml
中添加录音权限:
<uses-permission android:name="android.permission.RECORD_AUDIO"/>
其他可选参数
- 使用
FeatureActiveConfig
启用和禁用特定功能:
ChatView(
...
featureActiveConfig: FeatureActiveConfig(
enableSwipeToReply: true,
enableSwipeToSeeTime: false,
),
...
)
- 添加带有
ChatViewAppBar
的应用栏:
ChatView(
...
appBar: ChatViewAppBar(
profilePicture: profileImage,
chatTitle: "Simform",
userStatus: "online",
actions: [
Icon(Icons.more_vert),
],
),
...
)
- 添加带有
ChatBackgroundConfiguration
的消息列表配置:
ChatView(
...
chatBackgroundConfig: ChatBackgroundConfiguration(
backgroundColor: Colors.white,
backgroundImage: backgroundImage,
),
...
)
- 添加带有
SendMessageConfiguration
的发送消息配置:
ChatView(
...
sendMessageConfig: SendMessageConfiguration(
replyMessageColor: Colors.grey,
replyDialogColor:Colors.blue,
replyTitleColor: Colors.black,
closeIconColor: Colors.black,
),
...
)
- 添加带有
ChatBubbleConfiguration
的聊天气泡配置:
ChatView(
...
chatBubbleConfig: ChatBubbleConfiguration(
onDoubleTap: (){
// Your code goes here
},
outgoingChatBubbleConfig: ChatBubble( // 发送者的消息聊天气泡
color: Colors.blue,
borderRadius: const BorderRadius.only(
topRight: Radius.circular(12),
topLeft: Radius.circular(12),
bottomLeft: Radius.circular(12),
),
),
inComingChatBubbleConfig: ChatBubble( // 接收者的消息聊天气泡
color: Colors.grey.shade200,
borderRadius: const BorderRadius.only(
topLeft: Radius.circular(12),
topRight: Radius.circular(12),
bottomRight: Radius.circular(12),
),
),
)
...
)
- 添加带有
SwipeToReplyConfiguration
的滑动回复配置:
ChatView(
...
swipeToReplyConfig: SwipeToReplyConfiguration(
onLeftSwipe: (message, sendBy){
// Your code goes here
},
onRightSwipe: (message, sendBy){
// Your code goes here
},
),
...
)
- 添加带有
MessageConfiguration
的消息配置:
ChatView(
...
messageConfig: MessageConfiguration(
messageReactionConfig: MessageReactionConfiguration(), // 单个消息的表情反应配置
imageMessageConfig: ImageMessageConfiguration(
onTap: (){
// Your code goes here
},
shareIconConfig: ShareIconConfiguration(
onPressed: (){
// Your code goes here
},
),
),
),
...
)
- 添加带有
ReactionPopupConfiguration
的反应弹出配置:
ChatView(
...
reactionPopupConfig: ReactionPopupConfiguration(
backgroundColor: Colors.white,
userReactionCallback: (message, emoji){
// Your code goes here
}
padding: EdgeInsets.all(12),
shadow: BoxShadow(
color: Colors.black54,
blurRadius: 20,
),
),
...
)
- 添加带有
ReplyPopupConfiguration
的回复弹出配置:
ChatView(
...
replyPopupConfig: ReplyPopupConfiguration(
backgroundColor: Colors.white,
onUnsendTap:(message){ // message is 'Message' class instance
// Your code goes here
},
onReplyTap:(message){ // message is 'Message' class instance
// Your code goes here
},
onReportTap:(){
// Your code goes here
},
onMoreTap:(){
// Your code goes here
},
),
...
)
- 添加带有
RepliedMessageConfiguration
的回复消息配置:
ChatView(
...
repliedMessageConfig: RepliedMessageConfiguration(
backgroundColor: Colors.blue,
verticalBarColor: Colors.black,
repliedMsgAutoScrollConfig: RepliedMsgAutoScrollConfig(),
),
...
)
- 使用
TypeIndicatorConfiguration
自定义打字指示器:
ChatView(
...
typeIndicatorConfig: TypeIndicatorConfiguration(
flashingCircleBrightColor: Colors.grey,
flashingCircleDarkColor: Colors.black,
),
...
)
- 使用
ChatController.setTypingIndicator
显示/隐藏打字指示器:
/// 使用您的 [ChatController] 实例。
_chatContoller.setTypingIndicator = true; // 显示指示器
_chatContoller.setTypingIndicator = false; // 隐藏指示器
- 添加带有
LinkPreviewConfiguration
的链接预览配置:
ChatView(
...
chatBubbleConfig: ChatBubbleConfiguration(
linkPreviewConfig: LinkPreviewConfiguration(
linkStyle: const TextStyle(
color: Colors.white,
decoration: TextDecoration.underline,
),
backgroundColor: Colors.grey,
bodyStyle: const TextStyle(
color: Colors.grey.shade200,
fontSize:16,
),
titleStyle: const TextStyle(
color: Colors.black,
fontSize:20,
),
),
)
...
)
- 添加分页:
ChatView(
...
isLastPage: false,
featureActiveConfig: FeatureActiveConfig(
enablePagination: true,
),
loadMoreData: chatController.loadMoreData,
...
)
- 添加带有
ImagePickerIconsConfiguration
的图片选择器图标配置:
ChatView(
...
sendMessageConfig: SendMessageConfiguration(
imagePickerIconsConfig: ImagePickerIconsConfiguration(
cameraIconColor: Colors.black,
galleryIconColor: Colors.black,
)
)
...
)
- 添加
ChatViewState
自定义配置:
ChatView(
...
chatViewStateConfig: ChatViewStateConfiguration(
loadingWidgetConfig: ChatViewStateWidgetConfiguration(
loadingIndicatorColor: Colors.pink,
),
onReloadButtonTap: () {},
),
...
)
- 设置带有
RepliedMsgAutoScrollConfig
的自动滚动和高亮配置:
ChatView(
...
repliedMsgAutoScrollConfig: RepliedMsgAutoScrollConfig(
enableHighlightRepliedMsg: true,
highlightColor: Colors.grey,
highlightScale: 1.1,
)
...
)
- 当用户在
TextFieldConfiguration
中开始/停止输入时的回调:
ChatView(
...
sendMessageConfig: SendMessageConfiguration(
textFieldConfig: TextFieldConfiguration(
onMessageTyping: (status) {
// 发送打字/已完成状态给其他客户端
// your code goes here
},
/// 打字停止后,阈值时间在更改打字状态为 [TypeWriterStatus.typed] 之前。
/// 默认为1秒。
compositionThresholdTime: const Duration(seconds: 1),
),
...
)
)
- 传递自定义收据构建器或处理与收据相关的事宜,请参阅
ReceiptsWidgetConfig
在outgoingChatBubbleConfig
中:
ChatView(
...
featureActiveConfig: const FeatureActiveConfig(
/// 控制消息已读时间收据的可见性,默认为true
lastSeenAgoBuilderVisibility: false,
/// 控制消息 [receiptsBuilder] 的可见性
receiptsBuilderVisibility: false),
ChatBubbleConfiguration(
inComingChatBubbleConfig: ChatBubble(
onMessageRead: (message) {
/// 发送你的消息收据给其他客户端
debugPrint('Message Read');
},
),
outgoingChatBubbleConfig: ChatBubble(
receiptsWidgetConfig: ReceiptsWidgetConfig(
/// 自定义收据构建器
receiptsBuilder: _customReceiptsBuilder,
/// 是否在所有消息中显示收据,还是仅在最后一条消息中显示,类似于Instagram
showReceiptsIn: ShowReceiptsIn.lastMessage
),
),
),
...
)
如何使用
查看 博客 以获得更好的理解和基本实现。
此外,查看 example 目录中的 ‘example’ 应用,或者在 pub.dartlang.org 上的 ‘Example’ 标签,以获取完整的示例。
主要贡献者
Pranav Maid |
Vatsal Tanna |
Dhvanit Vaghani |
Ujas Majithiya |
许可证
MIT License
Copyright (c) 2022 Simform Solutions
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
更多关于Flutter聊天界面插件chatview2的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter聊天界面插件chatview2的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是一个关于如何在Flutter中使用chat_view2
插件来创建聊天界面的示例代码。chat_view2
是一个流行的Flutter插件,用于构建类似于聊天应用的UI。
首先,确保你已经在pubspec.yaml
文件中添加了chat_view2
依赖项:
dependencies:
flutter:
sdk: flutter
chat_view2: ^最新版本号 # 请替换为最新的版本号
然后运行flutter pub get
来安装依赖。
接下来,你可以按照以下步骤创建一个简单的聊天界面:
- 导入必要的包:
import 'package:flutter/material.dart';
import 'package:chat_view2/chat_view2.dart';
- 定义聊天消息数据模型:
class ChatMessage {
String sender;
String text;
DateTime timestamp;
bool isMe;
ChatMessage({required this.sender, required this.text, required this.timestamp, required this.isMe});
}
- 创建消息列表:
List<ChatMessage> messages = [
ChatMessage(sender: "Alice", text: "Hello!", timestamp: DateTime.now().subtract(Duration(minutes: 5)), isMe: false),
ChatMessage(sender: "Bob", text: "Hi Alice!", timestamp: DateTime.now().subtract(Duration(minutes: 4)), isMe: true),
ChatMessage(sender: "Alice", text: "How are you?", timestamp: DateTime.now().subtract(Duration(minutes: 3)), isMe: false),
// 更多消息...
];
- 构建聊天界面:
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: ChatScreen(messages: messages),
);
}
}
class ChatScreen extends StatelessWidget {
final List<ChatMessage> messages;
ChatScreen({required this.messages});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Chat'),
),
body: ChatView2(
messages: messages.map((message) => ChatMessageModel(
sender: message.sender,
text: message.text,
time: message.timestamp.toLocal().toString().split(' ')[1], // 格式化时间显示
isMe: message.isMe,
avatar: message.isMe ? 'assets/my_avatar.png' : 'assets/other_avatar.png', // 本地或网络图片URL
)).toList(),
padding: EdgeInsets.all(8.0),
messageTextStyle: TextStyle(fontSize: 16),
timeTextStyle: TextStyle(fontSize: 12, color: Colors.grey),
sendButton: FloatingActionButton(
onPressed: () {}, // 发送按钮点击事件
child: Icon(Icons.send),
backgroundColor: Colors.blue,
),
inputField: TextField(
decoration: InputDecoration(
border: InputBorder.none,
hintText: 'Type a message...',
),
),
alignment: CrossAxisAlignment.start,
),
);
}
}
在上面的代码中,我们定义了一个ChatMessage
类来表示聊天消息,并创建了一个消息列表。然后,在ChatScreen
中,我们使用ChatView2
小部件来渲染这些消息,并添加了一个发送按钮和输入框。
请注意:
- 你需要确保有
assets/my_avatar.png
和assets/other_avatar.png
这样的图片资源,或者你可以使用网络图片的URL。 timeTextStyle
和messageTextStyle
用于自定义时间和消息文本的样式。alignment
属性决定了消息是对齐到开始还是结束(对于RTL/LTR语言支持)。
这个示例代码展示了如何使用chat_view2
插件来创建一个基本的聊天界面。你可以根据需求进一步自定义和扩展这个界面。