Flutter文本超链接插件hyperlink_text的使用
Flutter文本超链接插件hyperlink_text的使用
Flutter组件设计用于显示带有可点击超链接的文本。它允许创建具有不同样式和行为的丰富文本。
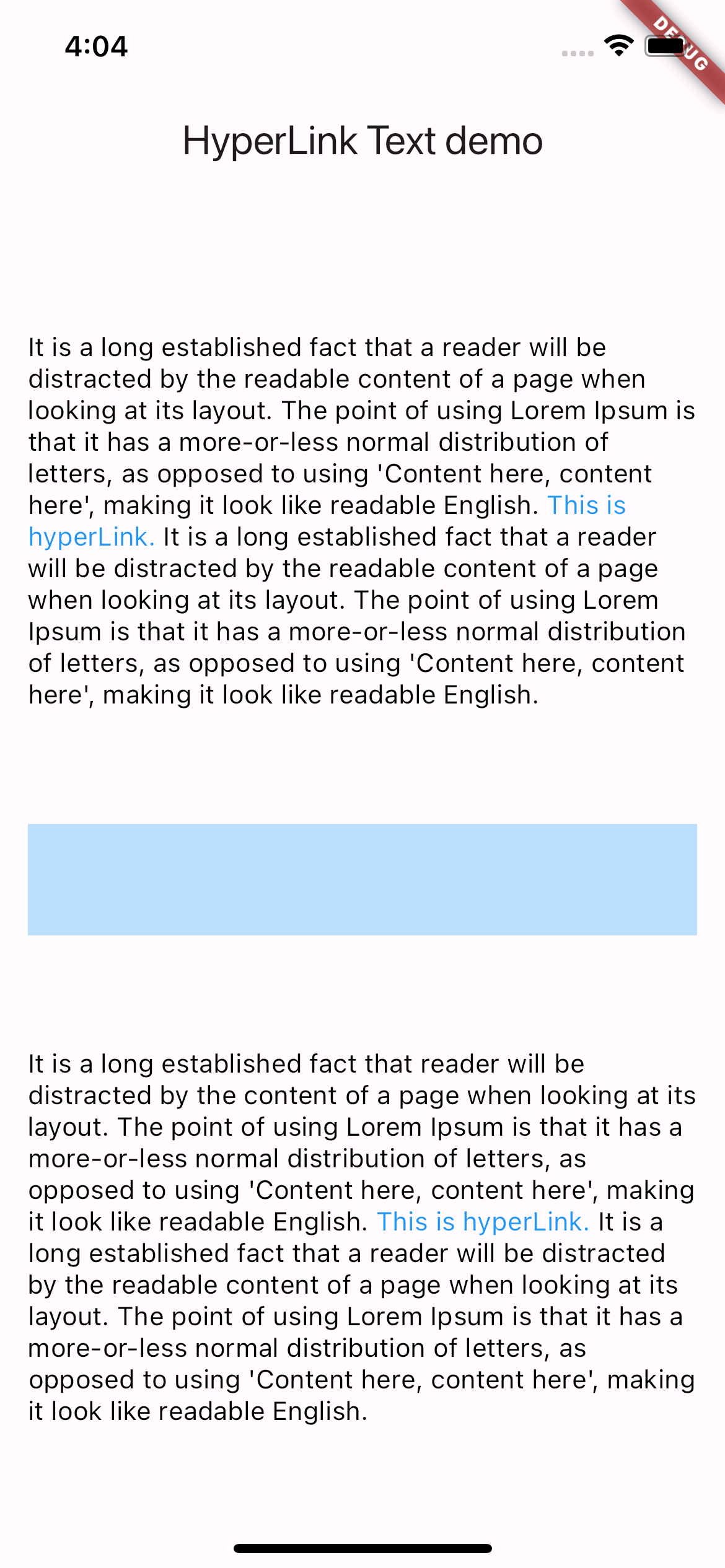
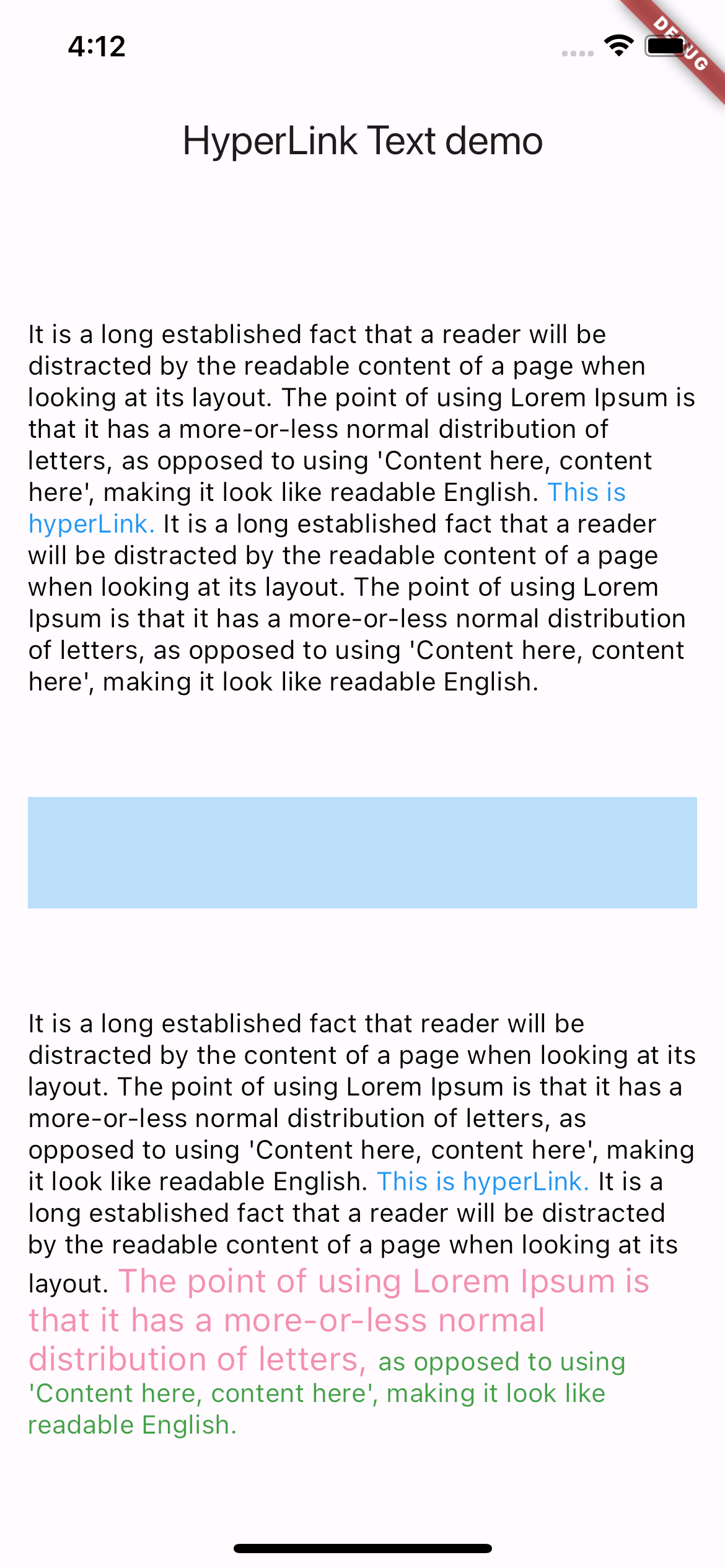
特性
- 显示带有可点击超链接的文本。
- 可以自定义普通文本和超链接的样式。
- 可以为单独的元素设置样式或使用全局样式。
- 支持创建具有不同样式和链接的丰富文本。
开始使用
- 在
pubspec.yaml
文件中添加包依赖:
dependencies:
hyperlink_text: ^latest
- 在Dart代码中导入包:
import 'package:hyperlink_text/hyperlink_text.dart';
使用方法
参考example
文件夹中的示例。
示例代码
import 'package:flutter/material.dart';
import 'package:hyperlink_text/Rich_text/rich_element.dart';
import 'package:hyperlink_text/hyperlink_text.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
title: 'HyperLink Text demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const MyHomePage(
title: 'HyperLink Text demo',
),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key, required this.title});
final String title;
[@override](/user/override)
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
[@override](/user/override)
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Padding(
padding: const EdgeInsets.all(15.0),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
HyperLinkText(
textStyle: const TextStyle(color: Colors.black),
hyperLinkStyle: const TextStyle(color: Colors.blue),
richElements: [
RichElement.text(
text:
"It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout. The point of using Lorem Ipsum is that it has a more-or-less normal distribution of letters, as opposed to using 'Content here, content here', making it look like readable English. ",
),
RichElement.link(
text: 'This is hyperLink. ',
url: 'https://google.com',
),
RichElement.text(
text:
"It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout. The point of using Lorem Ipsum is that it has a more-or-less normal distribution of letters, as opposed to using 'Content here, content here', making it look like readable English.",
),
]),
SizedBox(
height: 60,
width: double.maxFinite,
child: ColoredBox(
color: Colors.blue.shade100,
),
),
HyperLinkText(
textStyle: const TextStyle(color: Colors.black),
hyperLinkStyle: const TextStyle(color: Colors.blue),
richElements: [
RichElement.text(
text:
"It is a long established fact that reader will be distracted by the content of a page when looking at its layout. The point of using Lorem Ipsum is that it has a more-or-less normal distribution of letters, as opposed to using 'Content here, content here', making it look like readable English. ",
),
RichElement.link(
text: 'This is hyperLink. ',
url: 'https://google.com',
),
RichElement.text(
text:
"It is a long established fact that a reader will be distracted by the readable content of a page when looking at its layout. ",
),
RichElement.text(
text:
"The point of using Lorem Ipsum is that it has a more-or-less normal distribution of letters, ",
style:
TextStyle(fontSize: 18, color: Colors.pink.shade200)),
RichElement.text(
text:
"as opposed to using 'Content here, content here', making it look like readable English.",
style:
TextStyle(fontSize: 14, color: Colors.green.shade600))
])
],
),
),
);
}
}
更多关于Flutter文本超链接插件hyperlink_text的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
1 回复
更多关于Flutter文本超链接插件hyperlink_text的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是如何在Flutter中使用hyperlink_text
插件来实现文本超链接功能的代码示例。这个插件允许你在Flutter应用中显示和交互带有超链接的文本。
首先,确保你已经在pubspec.yaml
文件中添加了hyperlink_text
插件的依赖:
dependencies:
flutter:
sdk: flutter
hyperlink_text: ^x.y.z # 请替换为最新版本号
然后运行flutter pub get
来安装该插件。
接下来是一个简单的代码示例,展示了如何使用hyperlink_text
插件:
import 'package:flutter/material.dart';
import 'package:hyperlink_text/hyperlink_text.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Hyperlink Text Example'),
),
body: Center(
child: HyperlinkText(
text: "This is a link to Flutter's website: [Flutter](https://flutter.dev)",
onLinkTap: (link) {
// 在这里处理链接点击事件
// 例如,使用 Launcher 插件打开链接
if (await canLaunch(link)) {
await launch(link);
} else {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('Could not launch $link')),
);
}
},
// 自定义链接样式
linkStyle: TextStyle(color: Colors.blue, decoration: TextDecoration.underline),
// 自定义普通文本样式
textStyle: TextStyle(fontSize: 18),
),
),
),
);
}
}
// 引入用于打开链接的url_launcher插件
import 'package:url_launcher/url_launcher.dart';
// 检查是否可以打开链接的异步函数
Future<bool> canLaunch(String url) async {
if (await canLaunchUrl(Uri.parse(url))) {
return true;
} else if (await canLaunchUrl(Uri.parse('http://$url'))) {
return true;
} else if (await canLaunchUrl(Uri.parse('https://$url'))) {
return true;
} else {
return false;
}
}
解释
-
依赖项:在
pubspec.yaml
中添加hyperlink_text
和url_launcher
插件的依赖项。 -
导入包:在Dart文件中导入必要的包。
-
构建UI:
- 使用
HyperlinkText
小部件来显示包含超链接的文本。 text
参数定义了包含超链接的文本,其中链接部分用方括号括起来,并附上URL。onLinkTap
参数是一个回调函数,用于处理链接点击事件。在这个例子中,我们使用url_launcher
插件来打开链接。linkStyle
和textStyle
参数用于自定义链接和普通文本的样式。
- 使用
-
打开链接:
- 使用
canLaunch
函数检查是否可以打开链接。 - 如果可以,使用
launch
函数打开链接。 - 如果不能打开链接,显示一个SnackBar提示。
- 使用
请确保在实际项目中,根据需要调整URL格式和样式。这个示例提供了一个基本的框架,展示了如何使用hyperlink_text
插件来实现文本超链接功能。