Flutter设备管理插件my_device的使用
Flutter设备管理插件my_device的使用
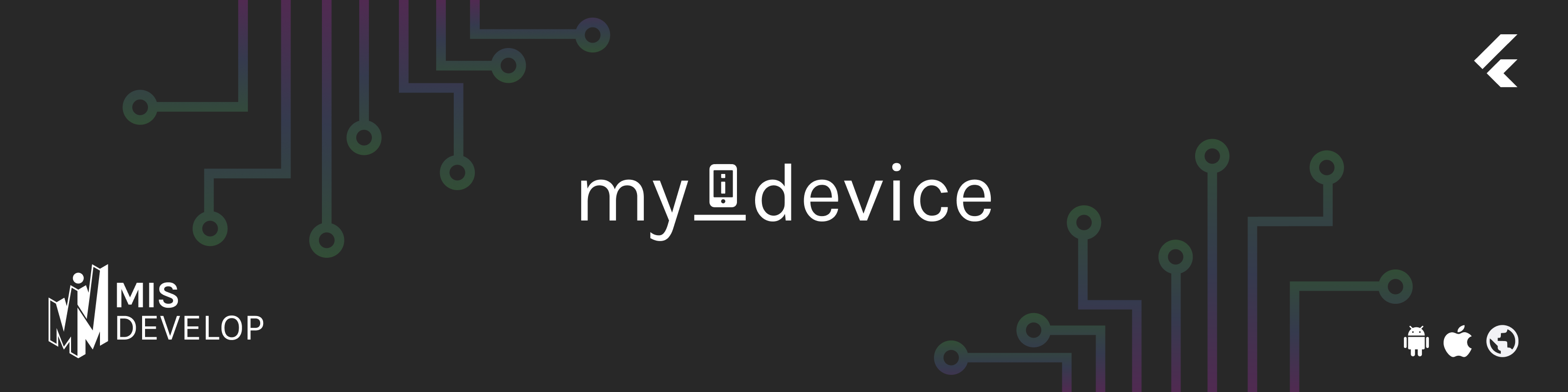
特性
敏捷且易于使用的设备信息处理工具 📱
包含 Device Info Plus ℹ️ 和 Connectivity Plus 🛜 实现。
开始使用
在你的 Flutter 项目的 pubspec.yaml
文件中添加以下依赖:
dependencies:
...
my_device: ^0.2.0
在你的库中添加以下导入:
import 'package:my_device/my_device.dart';
初始化上下文后,可以通过以下方式初始化 DeviceManager 实例:
DeviceManager(context);
你也可以使用配置方法来初始化 DeviceManager 实例、响应式断点和 Connectivity 配置:
DeviceManager.configure(
context: context,
responsiveBreakpoints: const ResponsiveBreakpoints(
mobile: 400, // 默认值为 500
desktop: 1000, // 默认值为 900
),
connectivityConfigure: ConnectivityConfigure(
onConnectivityChanged: (connectivity) {
print('Connectivity changed: $connectivity');
},
onConnectionLost: () {
print('Connection lost');
},
onConnectionReestablished: () {
print('Connection reestablished');
},
useDefaultBehaviour: true // 设置为 true 时会显示默认提示
),
);
// 移动视图 < 移动设备宽度
// 平板视图 > 移动设备宽度 && < 桌面设备宽度
// 桌面视图 > 桌面设备宽度
使用
任何方法都可以通过 DeviceManager 实例或通过 context
扩展访问。
获取屏幕高度和宽度
final screenHeight = DeviceManager.screenHeight;
final screenWidth = DeviceManager.screenWidth;
final height = DeviceManager.height;
final width = DeviceManager.width;
final heightFromContext = context.height;
final widthFromContext = context.width;
获取响应式尺寸(依赖于配置的响应式断点)
final isPhoneView = DeviceManager.isPhoneView; // 宽度 < 移动设备宽度
final isTabletView = DeviceManager.isTabletView; // 移动设备宽度 <= 宽度 < 桌面设备宽度
final isDesktopView = DeviceManager.isDesktopView; // 宽度 >= 桌面设备宽度
获取平台信息的方法
final isWeb = DeviceManager.isWeb;
final isAndroid = DeviceManager.isAndroid;
final isIOS = DeviceManager.isIOS;
final isMacOS = DeviceManager.isMacOS;
final isLinux = DeviceManager.isLinux;
final isWindows = DeviceManager.isWindows;
final platformName = DeviceManager.platformName;
final platformIcon = DeviceManager.platformIcon;
获取设备默认边距的方法
final padding = DeviceManager.viewPadding;
final paddingFromContext = context.viewPadding;
Connectivity Plus 方法
获取当前状态
final connectivity = DeviceManager.connectivity;
final connectivityFromContext = context.connectivity;
监听连接状态变化
DeviceManager.listenConnectivityChanges();
设置自定义连接状态方法(在配置回调之后)
DeviceManager.onConnectionLost = () {
print('Connection lost');
};
DeviceManager.onConnectionReestablished = () {
print('Connection reestablished');
};
DeviceManager.onConnectivityChanged = (connectivity) {
print('Connectivity changed: $connectivity');
};
Device Info Plus 缩写方法
final deviceInfo = DeviceManager.deviceInfo;
示例代码
以下是完整的示例代码:
import 'package:flutter/material.dart';
import 'package:my_device/my_device.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
title: 'My Device',
theme: ThemeData(
primarySwatch: Colors.deepPurple,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({
Key? key,
}) : super(key: key);
[@override](/user/override)
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
[@override](/user/override)
Widget build(BuildContext context) {
// 设置响应式断点
DeviceManager.configure(
context: context,
responsiveBreakpoints: const ResponsiveBreakpoints(
mobile: 500,
desktop: 1000,
),
connectivityConfigure: ConnectivityConfigure(
onConnectivityChanged: (connectivity) {
print('Connectivity changed: $connectivity');
},
onConnectionLost: () {
print('Connection lost');
},
onConnectionReestablished: () {
print('Connection reestablished');
},
),
);
// 或设置自定义函数(在配置之后)
DeviceManager.onConnectionLost = () {
print('Connection lost');
};
DeviceManager.onConnectionReestablished = () {
print('Connection reestablished');
};
DeviceManager.onConnectivityChanged = (connectivity) {
print('Connectivity changed: $connectivity');
};
// 获取给定上下文的 MediaQuery
final mediaQuery = DeviceManager.mediaQuery;
// 获取设备屏幕高度
final screenHeight = DeviceManager.screenHeight;
// 获取设备屏幕宽度
final screenWidth = DeviceManager.screenWidth;
// 也可以直接使用上下文获取宽度和高度
final width = context.width;
final height = context.height;
// 边缘垫片(物理铰链或岛屿)
var padding = DeviceManager.viewPadding;
return Scaffold(
appBar: AppBar(
title: Row(
mainAxisSize: MainAxisSize.min,
children: [
Text('My device - ${DeviceManager.platformName} '),
Icon(DeviceManager.platformIcon, color: Colors.black),
],
),
),
backgroundColor: const Color(0xFFc1c1c1),
body: Stack(
children: <Widget>[
Padding(padding: padding),
Flex(
direction: Axis.vertical,
children: [
Expanded(
flex: 0,
child: Padding(
padding: const EdgeInsets.all(4.0),
child: Card(
child: Text(
'Device Pixel Ratio: ' + mediaQuery.devicePixelRatio.toString(),
textAlign: TextAlign.center,
),
),
),
), // 像素比
Wrap(
alignment: WrapAlignment.center,
runAlignment: WrapAlignment.center,
spacing: 10,
runSpacing: 10,
children: [
SizedBox(
width: screenWidth / 2.3,
child: Card(
color: Colors.teal.withOpacity(.96),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(20)),
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
'Screen Width: ' + screenWidth.toString(),
textAlign: TextAlign.center,
style: const TextStyle(color: Colors.white),
),
Text(
'\nScreen Height: ' + screenHeight.toString(),
textAlign: TextAlign.center,
style: const TextStyle(color: Colors.white),
),
Text(
'\nDevice Orientation: ' + mediaQuery.orientation.toString(),
textAlign: TextAlign.center,
style: const TextStyle(color: Colors.white),
),
],
)),
), // 浅绿色容器
Column(
mainAxisSize: MainAxisSize.min,
children: [
SizedBox(
width: width / 2.3,
child: Card(
color: Colors.amber[700]!.withOpacity(.96),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(20)),
child: Text(
'Connectivity: ' + DeviceManager.connectivity.toString(),
textAlign: TextAlign.center,
style: const TextStyle(color: Colors.white),
),
),
),
SizedBox(
width: width / 2.3,
child: Card(
color: Colors.green[700]!.withOpacity(.96),
shape: RoundedRectangleBorder(borderRadius: BorderRadius.circular(20)),
child: Column(
children: [
Text(
'View type: ' +
(DeviceManager.isDesktopView
? 'Desktop'
: DeviceManager.isTabletView
? 'Tablet'
: 'Mobile'),
textAlign: TextAlign.center,
style: const TextStyle(color: Colors.white),
),
const Text(
"\nBreakpoints>>>\nDesktop: 1000\nMobile: 500",
textAlign: TextAlign.center,
style: TextStyle(color: Colors.white),
),
],
),
),
),
],
), // 黄色容器
],
), // 浅绿色 & 黄色容器
SizedBox(
height: height / 3,
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Card(
child: ListView(
children: [
displayEntry(DeviceManager.deviceInfo!),
],
)),
),
), // 设备信息
],
),
],
),
);
}
Widget displayEntry(Map e) {
List<Widget> aux = [];
for (var key in e.keys) {
aux.add(buildEntry(key, e[key]));
}
return Column(children: aux);
}
}
Widget buildEntry(key, e) {
if (e is! Map) {
if (e == null) {
return ListTile(
title: Text(key ?? ''),
);
}
return ListTile(
subtitle: Text(key ?? ''),
title: Text(e.toString()),
);
} else {
List<Widget> children = [];
for (var key in e.keys) {
children.add(buildEntry(key, e[key]));
}
return Column(children: children);
}
}
更多关于Flutter设备管理插件my_device的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter设备管理插件my_device的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,下面是一个关于如何在Flutter项目中使用my_device
插件来进行设备管理的示例代码。这个插件通常用于获取设备信息,例如品牌、型号、操作系统版本等。
首先,确保你已经在pubspec.yaml
文件中添加了my_device
依赖:
dependencies:
flutter:
sdk: flutter
my_device: ^x.y.z # 请替换为最新版本号
然后运行flutter pub get
来安装依赖。
接下来,你可以在你的Flutter项目中导入并使用my_device
插件。以下是一个完整的示例代码,展示如何获取设备信息并在UI中显示:
import 'package:flutter/material.dart';
import 'package:my_device/my_device.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Device Info Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: DeviceInfoScreen(),
);
}
}
class DeviceInfoScreen extends StatefulWidget {
@override
_DeviceInfoScreenState createState() => _DeviceInfoScreenState();
}
class _DeviceInfoScreenState extends State<DeviceInfoScreen> {
String _brand = 'Unknown';
String _model = 'Unknown';
String _osVersion = 'Unknown';
@override
void initState() {
super.initState();
_getDeviceInfo();
}
Future<void> _getDeviceInfo() async {
MyDevice myDevice = MyDevice();
DeviceInfo deviceInfo = await myDevice.getDeviceInfo();
setState(() {
_brand = deviceInfo.brand;
_model = deviceInfo.model;
_osVersion = deviceInfo.osVersion;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Device Info'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text('Brand:', style: TextStyle(fontSize: 18, fontWeight: FontWeight.bold)),
SizedBox(height: 8),
Text(_brand),
SizedBox(height: 16),
Text('Model:', style: TextStyle(fontSize: 18, fontWeight: FontWeight.bold)),
SizedBox(height: 8),
Text(_model),
SizedBox(height: 16),
Text('OS Version:', style: TextStyle(fontSize: 18, fontWeight: FontWeight.bold)),
SizedBox(height: 8),
Text(_osVersion),
],
),
),
);
}
}
在这个示例中,我们创建了一个简单的Flutter应用,该应用会显示设备的品牌、型号和操作系统版本。
- 导入必要的包:首先导入
flutter/material.dart
和my_device/my_device.dart
。 - 定义主应用:
MyApp
类作为应用的入口,设置了应用的标题和主题,并将DeviceInfoScreen
作为主页。 - 创建状态ful Widget:
DeviceInfoScreen
是一个状态ful widget,它会在初始化时调用_getDeviceInfo
方法来获取设备信息。 - 获取设备信息:在
_getDeviceInfo
方法中,我们实例化了MyDevice
类并调用了getDeviceInfo
方法来获取设备信息,然后更新状态以反映这些信息。 - 显示设备信息:在
build
方法中,我们构建了一个简单的UI来显示设备信息。
请注意,实际使用时,MyDevice
类和getDeviceInfo
方法的调用可能会因插件版本的不同而有所变化。因此,建议查阅my_device
插件的官方文档以获取最新的使用方法和API参考。