Flutter支付集成插件stone_payments的使用
Flutter支付集成插件stone_payments的使用
本插件是一个为Flutter开发提供的集成包,用于与Stone公司的支付机器进行交互。通过该插件,您可以轻松地在您的Flutter应用中处理支付事务。
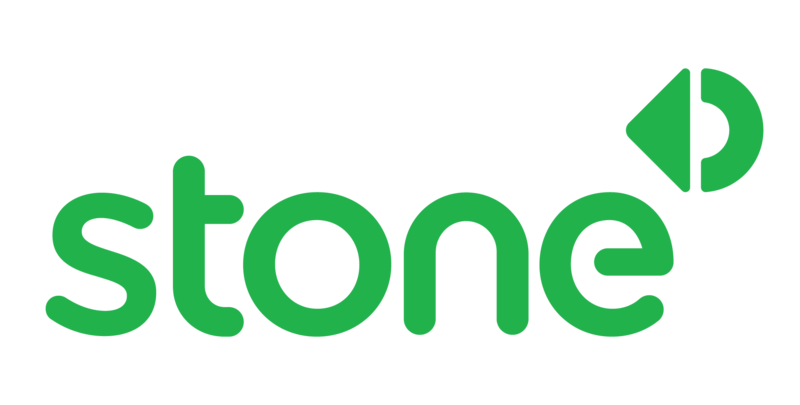
安装
要使用此插件,您需要将stone_payments
作为依赖项添加到您的pubspec.yaml
文件中:
dependencies:
stone_payments: ^0.1.7
然后运行flutter pub get
以安装插件。
在项目的根目录下的local.properties
文件中添加以下变量,并替换为您自己的token:
packageCloudReadToken=您的Token
确保您的minSdkVersion
设置为23或更高:
minSdkVersion=23
注意!
支持的设备
- Positivo - L400
- Positivo - L300
- Ingenico - APOS A8
- Sunmi - P2
- Gertec - GPOS700X
使用
要在您的Flutter应用中使用Stone支付机器的集成,您需要遵循以下步骤:
1. 激活支付机器
await StonePayments.activateStone(
appName: '我的应用',
stoneCode: '12345678', // 从Stone团队获取的代码
qrCodeProviderId: 'PROVIDER_ID', // 如果您将使用PIX,请提供由Stone集成团队提供的QR_CODE_PROVIDER_ID
qrCodeAuthentication: 'TOKEN', // 如果您将使用PIX,请提供由Stone集成团队提供的QR_CODE_AUTHENTICATION
);
2. 开始支付交易
final transactionResult = await StonePayments.transaction(
valor: 5,
typeTransaction: TypeTransactionEnum.debit,
parcelas: 1, // 可选参数
printReceipt: false, // 可选参数:打印商户收据
onPixQrCode: (value) {
setState(() {
image = base64Decode(value);
});
}, // 可选参数:接收PIX二维码的回调函数
);
transaction
方法返回一个包含交易结果的Future<Transaction>
对象,其中Transaction
是一个具有以下属性的对象:
class Transaction {
final String? acquirerTransactionKey;
final String? actionCode;
final String? aid;
final String? amount;
final String? appLabel;
final String? arcq;
final String? authorizationCode;
final bool? capture;
final String? cardBrand;
final int? cardBrandId;
final String? cardBrandName;
final String? cardExpireDate;
final String? cardHolderName;
final String? cardHolderNumber;
final String? cardSequenceNumber;
final String? commandActionCode;
final String? date;
final String? entryMode;
final String? iccRelatedData;
final int? idFromBase;
final String? initiatorTransactionKey;
final String? instalmentTransaction;
final String? instalmentType;
final bool? isFallbackTransaction;
final List<String>? qualifiers;
final String? saleAffiliationKey;
final String? serviceCode;
final String? time;
final String? timeToPassTransaction;
final TransAppSelectedInfo? transAppSelectedInfo;
final String? transactionReference;
final String? transactionStatus;
final String? typeOfTransactionEnum;
}
class TransAppSelectedInfo {
final String? aid;
final int? brandId;
final String? brandName;
final String? cardAppLabel;
final String? paymentBusinessModel;
final TransactionTypeInfo? transactionTypeInfo;
}
class TransactionTypeInfo {
final String? appLabel;
final int? id;
final String? transTypeEnum;
}
3. 获取交易状态
StonePayments.onMessageListener((mensagem) {
setState(() {
texto = mensagem;
});
});
4. 打印文本和图像
需要传递一个包含ItemPrintModel
的列表给print
方法,其中列表中的每个项目都是收据的一行,可以是文本或base64编码的图像。
base64编码的图像只能有最大尺寸为380x595像素,如Stone文档所述。
await StonePayments.print([
const ItemPrintModel(
type: ItemPrintTypeEnum.text,
data: '测试标题',
),
const ItemPrintModel(
type: ItemPrintTypeEnum.text,
data: '测试副标题',
),
ItemPrintModel(
type: ItemPrintTypeEnum.image,
data: imgBase64,
),
]);
示例
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await StonePayments.activateStone(
appName: '我的应用',
stoneCode: '12345678',
qrCodeAuthorization: 'TOKEN',
qrCodeProviderId: 'PROVIDER_ID',
);
runApp(const MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
[@override](/user/override)
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
String text = '正在运行';
late StreamSubscription<String> listen;
Uint8List? image;
[@override](/user/override)
void initState() {
listen = StonePayments.onMessageListener((message) {
setState(() {
text = message;
});
});
super.initState();
}
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('插件示例应用'),
),
body: SizedBox(
width: double.infinity,
child: SingleChildScrollView(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text(text),
ElevatedButton(
onPressed: () async {
if (listen.isPaused) {
listen.resume();
}
try {
final result = await StonePayments.transaction(
value: 5,
typeTransaction: TypeTransactionEnum.pix,
onPixQrCode: (value) {
setState(() {
image = base64Decode(value);
});
},
);
print(result);
} catch (e) {
listen.pause();
setState(() {
text = "支付失败";
});
}
},
child: const Text('购买 R$5.00'),
),
if (image != null)
Image.memory(
image!,
height: 200,
),
Image.asset('assets/flutter5786.png'),
ElevatedButton(
onPressed: () async {
try {
var byteData = await rootBundle.load('assets/flutter5786.png');
var imgBase64 = base64Encode(byteData.buffer.asUint8List());
var items = [
const ItemPrintModel(
type: ItemPrintTypeEnum.text,
data: '测试标题',
),
const ItemPrintModel(
type: ItemPrintTypeEnum.text,
data: '测试副标题',
),
ItemPrintModel(
type: ItemPrintTypeEnum.image,
data: imgBase64,
),
];
await StonePayments.print(items);
} catch (e) {
setState(() {
text = "打印失败";
});
}
},
child: const Text('打印'),
),
ElevatedButton(
onPressed: () async {
try {
await StonePayments.printReceipt(TypeOwnerPrintEnum.merchant);
} catch (e) {
setState(() {
text = "打印失败";
});
}
},
child: const Text('打印商户副本'),
),
ElevatedButton(
onPressed: () async {
try {
await StonePayments.printReceipt(TypeOwnerPrintEnum.client);
} catch (e) {
setState(() {
text = "打印失败";
});
}
},
child: const Text('打印客户副本'),
),
],
),
),
),
),
);
}
}
更多关于Flutter支付集成插件stone_payments的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter支付集成插件stone_payments的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是一个关于如何在Flutter项目中集成并使用stone_payments
插件进行支付的示例代码。这个插件假设是用于处理支付事务的,但请注意,具体的API和集成细节可能会根据插件的版本和Stone Payments的实际API有所变化。以下代码仅供参考,并可能需要根据你的具体需求进行调整。
首先,确保你已经在pubspec.yaml
文件中添加了stone_payments
依赖:
dependencies:
flutter:
sdk: flutter
stone_payments: ^latest_version # 请替换为实际的最新版本号
然后,运行flutter pub get
来安装依赖。
接下来,在你的Flutter项目中,你可以按照以下步骤使用stone_payments
插件进行支付集成:
-
初始化插件:
在你的主文件(例如
main.dart
)或者需要初始化支付的页面,导入stone_payments
包并进行初始化。import 'package:flutter/material.dart'; import 'package:stone_payments/stone_payments.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Stone Payments Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: PaymentScreen(), ); } } class PaymentScreen extends StatefulWidget { @override _PaymentScreenState createState() => _PaymentScreenState(); } class _PaymentScreenState extends State<PaymentScreen> { StonePayments? _stonePayments; @override void initState() { super.initState(); // 初始化StonePayments实例,这里需要传入你的API密钥或其他必要的配置 _stonePayments = StonePayments( publicKey: 'your_public_key_here', // 替换为你的公钥 environment: StoneEnvironment.sandbox, // 使用沙盒环境,生产环境请改为StoneEnvironment.production ); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Stone Payments Demo'), ), body: Center( child: ElevatedButton( onPressed: () async { // 调用支付方法 await _initiatePayment(); }, child: Text('Initiate Payment'), ), ), ); } Future<void> _initiatePayment() async { try { // 构建支付请求,这里需要根据你的业务需求填充支付信息 var paymentRequest = StonePaymentRequest( amount: 1000, // 金额,单位通常是分(例如1000代表10.00美元) currency: 'USD', // 货币代码 description: 'Test Payment', // 支付描述 // 其他必要的支付信息,如card信息、持卡人信息等,这里为了简化省略 ); // 发起支付请求 var paymentResponse = await _stonePayments!.createPayment(paymentRequest); // 处理支付响应 if (paymentResponse.isSuccess) { print('Payment successful: ${paymentResponse.data}'); } else { print('Payment failed: ${paymentResponse.errorMessage}'); } } catch (e) { print('Error initiating payment: $e'); } } }
-
处理支付响应:
在上面的代码中,
_initiatePayment
方法负责构建支付请求并调用_stonePayments!.createPayment
方法发起支付。支付响应通过paymentResponse
对象获取,你可以根据响应结果进行相应的处理。 -
注意事项:
- 确保你已经从Stone Payments获取了必要的API密钥,并且已经在Stone Payments后台配置了正确的支付信息。
- 在生产环境中,请确保将
environment
设置为StoneEnvironment.production
。 - 根据你的业务需求,支付请求
StonePaymentRequest
可能需要包含更多的信息,如持卡人姓名、卡号、有效期、CVV等。这些信息通常通过UI表单收集,并在发起支付请求时传递给插件。
请注意,由于stone_payments
插件的具体API和实现细节可能随版本变化,因此上述代码可能需要根据你使用的插件版本进行调整。此外,实际支付集成过程中可能需要处理更多的安全性和用户体验方面的考虑,如支付结果的UI展示、错误处理等。建议详细阅读插件的官方文档和示例代码,以确保正确集成和使用。