Flutter侧边栏导航插件sidebar_bigeagle的使用
Flutter侧边栏导航插件sidebar_bigeagle的使用
简介
sidebar_bigeagle
是一个用于添加方便的侧边栏的小部件,适用于Web和桌面应用。
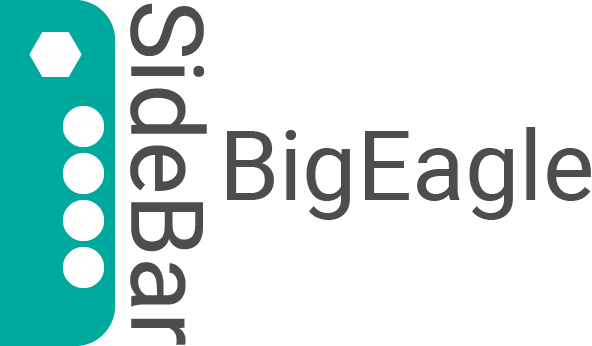
Web示例
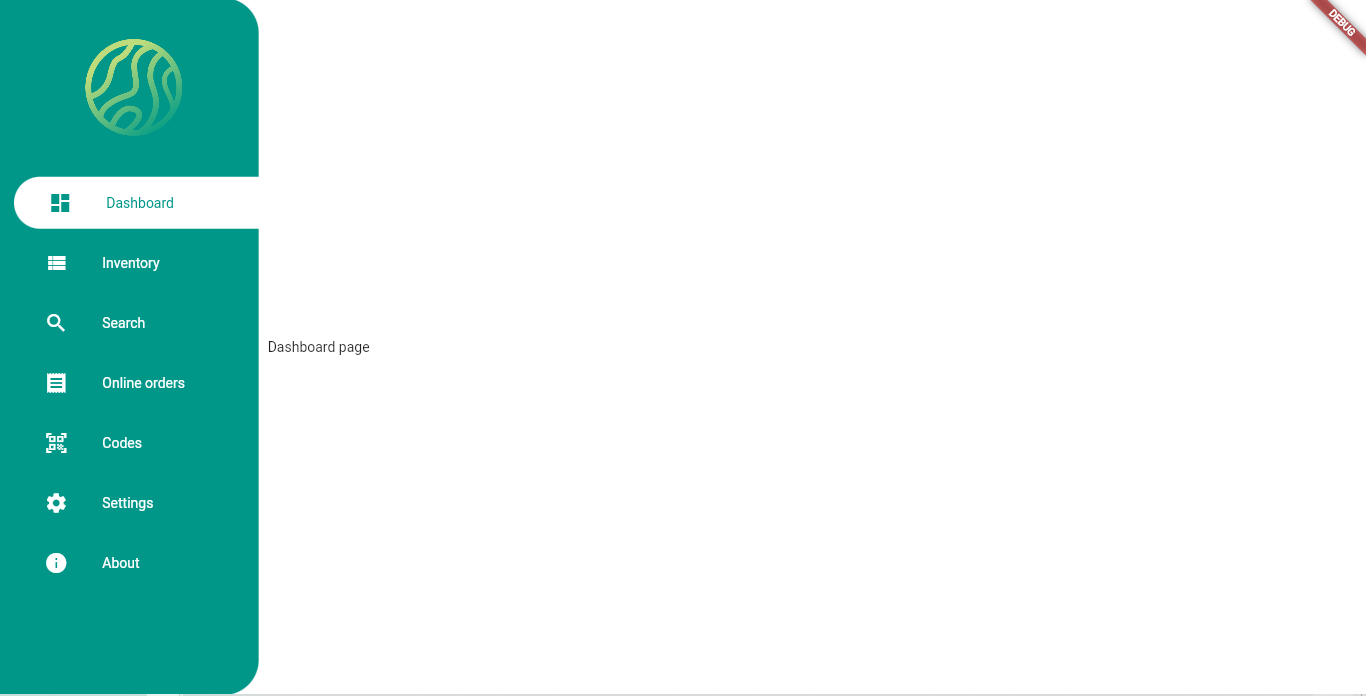
移动Web示例
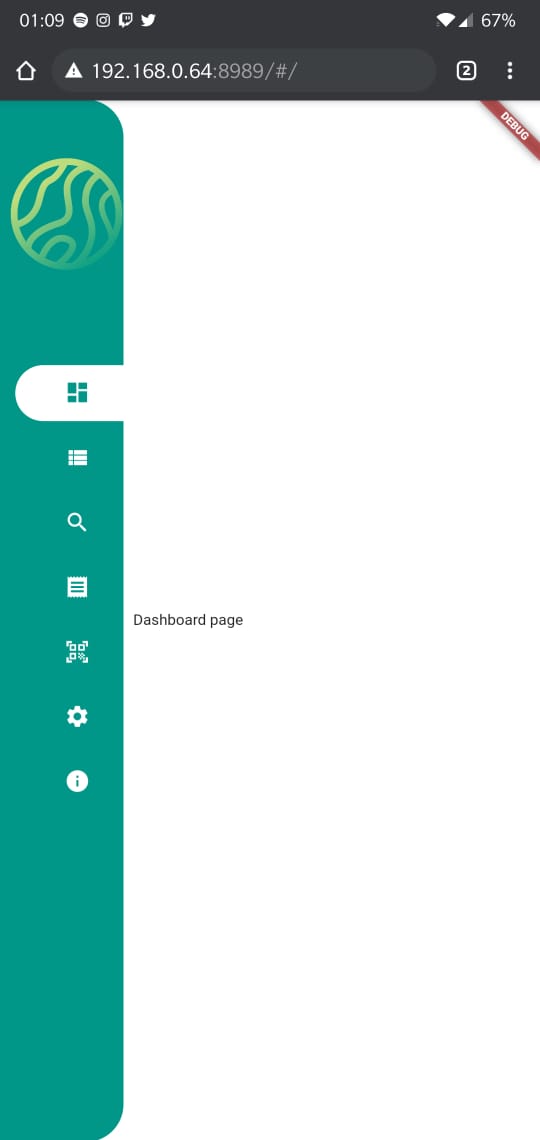
作者及贡献
该插件由Augusto Cabrera开发。您可以访问他的网站查看所有作品。
捐赠
如果您喜欢此插件并希望支持开发者,可以通过以下方式捐赠:
- PayPal
- 比特币地址: 1Fne5WswXHXrYXozkwNAMLayWyxVfZGbMy
完整示例代码
import 'package:flutter/material.dart';
import 'package:sidebar_bigeagle/sidebar_bigeagle.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
[@override](/user/override)
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
int _selectedIndex = 0; // 当前选中的页面索引,默认为0
List<Widget> pages = []; // 存储应用内容的页面列表
[@override](/user/override)
void initState() {
super.initState();
pages = [
// 页面列表,这是主应用内容
Text("仪表板页面"),
Text("库存页面"),
Text("搜索页面"),
Text("在线订单页面"),
Text("代码页面"),
Text("设置页面"),
Text("关于页面"),
];
}
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('BigEagle侧边栏示例应用'),
),
body: Row(
children: [
// SideBar Big Eagle
SideBar(
color: Colors.teal, // 侧边栏颜色
appColor: Colors.white, // 应用背景色,默认为白色
accentColor: Colors.white, // 选中按钮的颜色,默认为白色
onHoverScale: 1.2, // 鼠标悬停时按钮缩放比例,建议值为1.2,最小值为0.5,最大值为2
borderRadius: 35.0, // 边框圆角半径,默认为35.0
elevation: 6.0, // 阴影高度,默认为0.0
children: [
// 按钮列表
SideBarButtonFlat(title: "仪表板", icon: Icons.dashboard),
SideBarButtonFlat(title: "库存", icon: Icons.view_list),
SideBarButtonFlat(title: "搜索", icon: Icons.search),
SideBarButtonFlat(title: "在线订单", icon: Icons.receipt),
SideBarButtonFlat(title: "代码", icon: Icons.qr_code_scanner),
SideBarButtonFlat(title: "设置", icon: Icons.settings),
SideBarButtonFlat(title: "关于", icon: Icons.info),
],
onChange: (value) {
// 这是在按钮被点击时调用的回调函数
setState(() {
_selectedIndex = value;
});
},
),
// 主内容区域
Expanded(
child: pages[_selectedIndex],
)
],
),
),
);
}
}
更多关于Flutter侧边栏导航插件sidebar_bigeagle的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter侧边栏导航插件sidebar_bigeagle的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是如何在Flutter项目中使用sidebar_bigeagle
插件来实现侧边栏导航的示例代码。sidebar_bigeagle
是一个流行的侧边栏导航插件,用于构建具有抽屉式导航菜单的应用。
首先,确保你已经在pubspec.yaml
文件中添加了sidebar_bigeagle
依赖:
dependencies:
flutter:
sdk: flutter
sidebar_bigeagle: ^最新版本号 # 替换为最新版本号
然后运行flutter pub get
来安装依赖。
接下来,是一个完整的示例代码,展示如何使用sidebar_bigeagle
来实现侧边栏导航:
import 'package:flutter/material.dart';
import 'package:sidebar_bigeagle/sidebar_bigeagle.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Sidebar Bigeagle Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MainScreen(),
);
}
}
class MainScreen extends StatefulWidget {
@override
_MainScreenState createState() => _MainScreenState();
}
class _MainScreenState extends State<MainScreen> with SingleTickerProviderStateMixin {
SidebarController _sidebarController;
@override
void initState() {
super.initState();
_sidebarController = SidebarController();
}
@override
Widget build(BuildContext context) {
return Scaffold(
drawer: Sidebar(
controller: _sidebarController,
header: Container(
height: 150,
decoration: BoxDecoration(color: Colors.blue),
child: Center(
child: Text(
'Drawer Header',
style: TextStyle(color: Colors.white, fontSize: 24),
),
),
),
items: [
SidebarItem(
icon: Icons.home,
title: 'Home',
onTap: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => HomePage()),
);
_sidebarController.close();
},
),
SidebarItem(
icon: Icons.settings,
title: 'Settings',
onTap: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SettingsPage()),
);
_sidebarController.close();
},
),
// 添加更多侧边栏项
],
),
appBar: AppBar(
title: Text('Main Screen'),
leading: IconButton(
icon: Icon(Icons.menu),
onPressed: () => _sidebarController.toggle(),
),
),
body: Center(
child: Text('Welcome to the Main Screen!'),
),
);
}
}
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Home Page'),
),
body: Center(
child: Text('You are on the Home Page!'),
),
);
}
}
class SettingsPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Settings Page'),
),
body: Center(
child: Text('You are on the Settings Page!'),
),
);
}
}
代码说明:
- 依赖引入:确保在
pubspec.yaml
中添加了sidebar_bigeagle
依赖。 - MainApp:主应用类,配置MaterialApp并设置主页为
MainScreen
。 - MainScreen:主页类,使用
Sidebar
组件创建侧边栏导航菜单。SidebarController
:用于控制侧边栏的打开和关闭。Sidebar
:侧边栏组件,包括头部和侧边栏项。SidebarItem
:侧边栏项,每个项包括图标、标题和点击事件。
- HomePage 和 SettingsPage:两个简单的页面,用于导航到不同的内容。
运行应用:
确保你已经正确安装了依赖,然后运行应用。你应该能够看到一个带有侧边栏导航的主屏幕。点击侧边栏项将导航到相应的页面。
这个示例展示了如何使用sidebar_bigeagle
插件来创建一个简单的侧边栏导航应用。根据你的需求,你可以进一步自定义和扩展这个示例。