Flutter谷歌应用商店更新提示插件in_googleplay_update的使用
Flutter谷歌应用商店更新提示插件in_googleplay_update的使用
Presented by
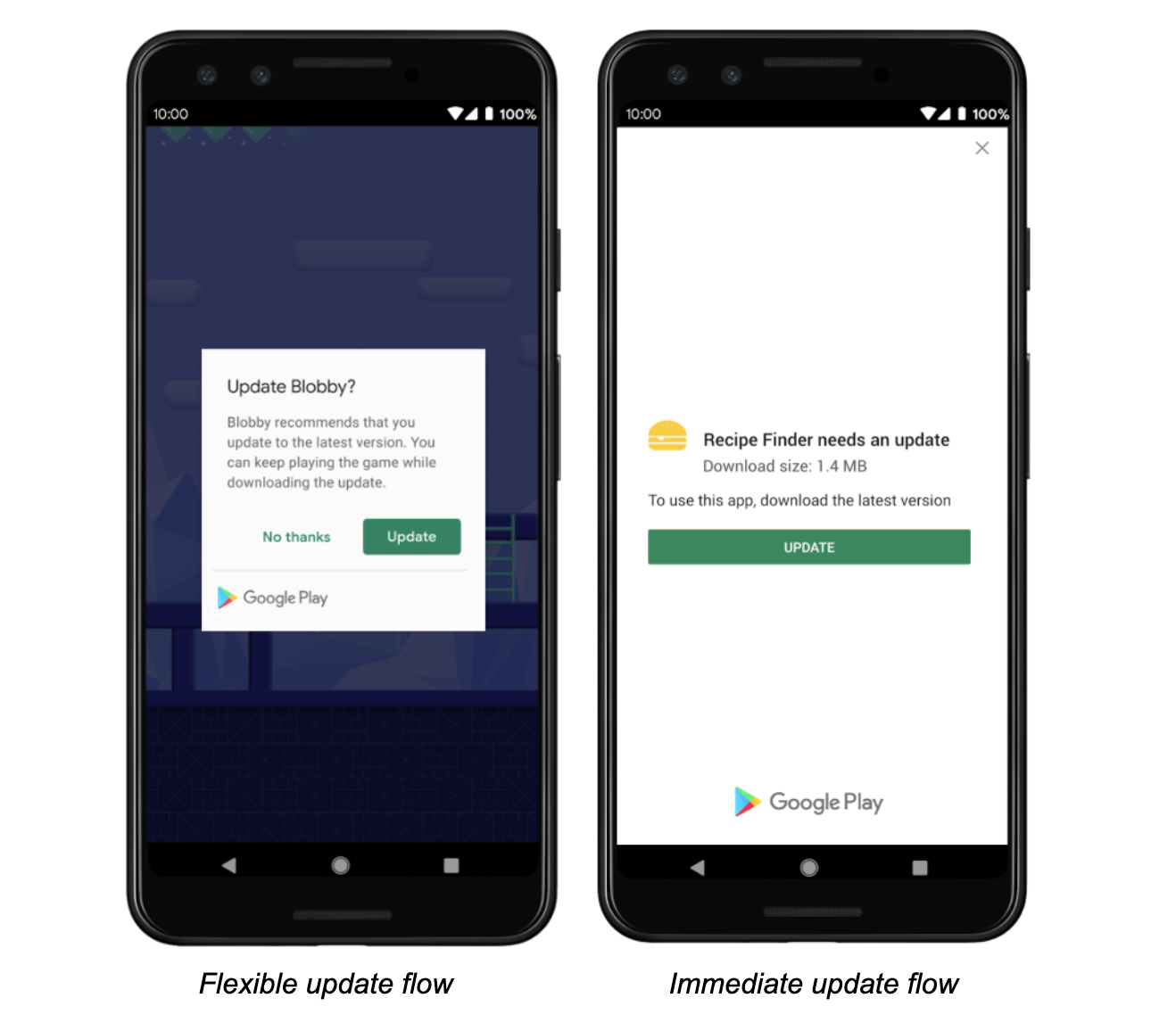
Maintained by ACHMAD MAULANA
in_googleplay_update
in_googleplay_update简介
in_googleplay_update
是一个用于在 Android 应用中实现 Google Play 应用商店更新功能的 Flutter 插件。它通过调用官方的 Android API 来完成应用内的即时更新或灵活更新。
官方文档:
https://developer.android.com/guide/app-bundle/in-app-updates
使用方法
该插件提供了以下方法:
-
检查是否有更新可用
Future<AppUpdateInfo> checkForUpdate()
检查是否有新的版本可用。
-
立即执行更新
Future<void> performImmediateUpdate()
执行即时更新(全屏模式)。
-
开始灵活更新
Future<void> startFlexibleUpdate()
开始后台下载的灵活更新。
-
完成灵活更新
Future<void> completeFlexibleUpdate()
安装已下载的灵活更新。
-
监听灵活更新进度
Stream<String> flexibleUpdateStream()
返回 JSON 字符串,包含已下载字节数和总字节数:
{"bytes_downloaded": "string", "total_bytes_to_download": "string"}
可用于展示下载进度条。
完整示例代码
以下是一个完整的示例代码,展示了如何使用 in_googleplay_update
插件进行更新检查和进度展示。
import 'dart:async';
import 'dart:convert';
import 'dart:developer';
import 'package:flutter/material.dart';
import 'package:in_googleplay_update/in_googleplay_update.dart';
import 'package:package_info_plus/package_info_plus.dart';
import 'package:filesize/filesize.dart';
void main() => runApp(MyApp());
class MyApp extends StatefulWidget {
[@override](/user/override)
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
PackageInfo _packageInfo = PackageInfo(
appName: 'Unknown',
packageName: 'Unknown',
version: 'Unknown',
buildNumber: 'Unknown',
buildSignature: 'Unknown',
installerStore: 'Unknown',
);
[@override](/user/override)
void initState() {
super.initState();
_initPackageInfo();
}
Future<void> _initPackageInfo() async {
final info = await PackageInfo.fromPlatform();
setState(() {
_packageInfo = info;
});
}
Widget _infoTile(String title, String subtitle) {
return ListTile(
title: Text(title),
subtitle: Text(subtitle.isEmpty ? 'Not set' : subtitle),
);
}
AppUpdateInfo? _updateInfo;
AppUpdateResult? _updateResult;
GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey();
bool _flexibleUpdateAvailable = false;
Future<void> checkForUpdate() async {
InAppPlayUpdate.checkForUpdate().then((info) {
setState(() {
_updateInfo = info;
});
}).catchError((e) {
showSnack(e.toString());
});
}
void showSnack(String text) {
if (_scaffoldKey.currentState != null) {
ScaffoldMessenger.of(_scaffoldKey.currentState!).showSnackBar(
SnackBar(content: Text(text)),
);
}
}
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
key: _scaffoldKey,
appBar: AppBar(
title: const Text('Google Play 更新示例'),
),
body: SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Column(
children: [
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
_infoTile('更新可用性', '${_updateInfo?.updateAvailability}'),
_infoTile('立即更新允许', '${_updateInfo?.immediateUpdateAllowed}'),
_infoTile('可用版本号', '${_updateInfo?.availableVersionCode}'),
_infoTile('安装状态', '${_updateInfo?.installStatus}'),
_infoTile('包名', '${_updateInfo?.packageName}'),
_infoTile('客户端版本过期天数', '${_updateInfo?.clientVersionStalenessDays}'),
_infoTile('更新优先级', '${_updateInfo?.updatePriority}'),
_infoTile('更新结果', '${_updateResult}'),
],
),
ElevatedButton(
child: const Text('检查更新'),
onPressed: () => checkForUpdate(),
),
ElevatedButton(
child: Text('立即更新'),
onPressed: _updateInfo?.updateAvailability == UpdateAvailability.updateAvailable
? () {
InAppPlayUpdate.performImmediateUpdate().catchError(
(e) => showSnack(e.toString()),
);
}
: null,
),
ElevatedButton(
child: Text('开始灵活更新'),
onPressed: _updateInfo?.updateAvailability == UpdateAvailability.updateAvailable
? () {
InAppPlayUpdate.startFlexibleUpdate().then((_) {
setState(() {
_updateResult = _;
_flexibleUpdateAvailable = true;
});
}).catchError(
(e) => showSnack(e.toString()),
);
}
: null,
),
StreamBuilder<String>(
stream: InAppPlayUpdate.flexibleUpdateStream(),
builder: (context, snapshot) {
if (snapshot.hasData) {
String byteStringDownload = snapshot.data ?? '{}';
final byteDownload = json.decode(byteStringDownload);
return Column(
children: [
if (int.parse(byteDownload['bytes_downloaded']) == 0 &&
int.parse(byteDownload['total_bytes_to_download']) == 0) ...[
ElevatedButton(
onPressed: () {
InAppPlayUpdate.completeFlexibleUpdate().then((_) {
showSnack("成功!");
}).catchError(
(e) => showSnack(e.toString()),
);
},
child: const Text('完成灵活更新'),
),
] else ...[
LinearProgressIndicator(
value: (int.parse(byteDownload['bytes_downloaded']) /
int.parse(byteDownload['total_bytes_to_download'])),
),
Text(
'${filesize(int.parse(byteDownload['bytes_downloaded']))}/${filesize(int.parse(byteDownload['total_bytes_to_download']))}',
style: const TextStyle(fontSize: 14),
)
]
],
);
} else {
return Column(
children: [
SizedBox(height: 10),
SizedBox(
width: 30,
height: 30,
child: CircularProgressIndicator(),
),
Text(
'加载中...',
style: const TextStyle(fontSize: 14),
)
],
);
}
},
),
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
_infoTile('应用名称', _packageInfo.appName),
_infoTile('包名', _packageInfo.packageName),
_infoTile('版本号', _packageInfo.version),
_infoTile('构建号', _packageInfo.buildNumber),
_infoTile('签名', _packageInfo.buildSignature),
_infoTile(
'安装来源',
_packageInfo.installerStore ?? '未知',
),
],
),
],
),
),
),
),
);
}
}
更多关于Flutter谷歌应用商店更新提示插件in_googleplay_update的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter谷歌应用商店更新提示插件in_googleplay_update的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
in_googleplay_update
是一个 Flutter 插件,用于检查 Google Play 商店中的应用更新,并在有新版本时提示用户进行更新。这个插件可以帮助开发者确保用户始终使用最新版本的应用,从而获得最佳体验和最新的功能。
安装插件
首先,你需要在 pubspec.yaml
文件中添加 in_googleplay_update
插件的依赖:
dependencies:
flutter:
sdk: flutter
in_googleplay_update: ^1.0.0 # 请使用最新版本
然后运行 flutter pub get
来安装插件。
使用插件
-
导入插件
在你的 Dart 文件中导入插件:
import 'package:in_googleplay_update/in_googleplay_update.dart';
-
检查更新
你可以在应用的某个地方(例如在
initState
中)调用checkForUpdate
方法来检查是否有新版本:void checkForAppUpdate() async { try { final updateInfo = await InGoogleplayUpdate.checkForUpdate(); if (updateInfo.updateAvailability == UpdateAvailability.updateAvailable) { // 如果有新版本,提示用户更新 showUpdateDialog(updateInfo); } } catch (e) { print("Error checking for update: $e"); } }
-
显示更新提示
如果检测到新版本,你可以显示一个对话框提示用户更新:
void showUpdateDialog(UpdateInfo updateInfo) { showDialog( context: context, builder: (BuildContext context) { return AlertDialog( title: Text("New Update Available"), content: Text("A new version of the app is available. Please update to continue."), actions: <Widget>[ TextButton( child: Text("Update"), onPressed: () { // 打开 Google Play 商店进行更新 InGoogleplayUpdate.launchUpdate(); Navigator.of(context).pop(); }, ), TextButton( child: Text("Later"), onPressed: () { Navigator.of(context).pop(); }, ), ], ); }, ); }
-
处理更新流程
你可以根据
updateInfo
中的信息来决定如何处理更新流程。例如,如果updateInfo.immediateUpdateAllowed
为true
,你可以直接启动更新流程,而不需要用户确认。if (updateInfo.immediateUpdateAllowed) { InGoogleplayUpdate.launchUpdate(); } else { showUpdateDialog(updateInfo); }
注意事项
-
Google Play 商店的限制:
in_googleplay_update
插件依赖于 Google Play 商店的更新机制,因此它只能在通过 Google Play 商店分发的应用中使用。 -
测试:在开发过程中,你可能无法直接测试更新流程,因为 Google Play 商店通常不会在开发版本中提供更新。你可以通过发布一个测试版本来验证更新流程。
-
权限:确保你的应用具有访问网络的权限,因为插件需要与 Google Play 商店进行通信。
示例代码
以下是一个完整的示例代码,展示了如何在 Flutter 应用中使用 in_googleplay_update
插件:
import 'package:flutter/material.dart';
import 'package:in_googleplay_update/in_googleplay_update.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
home: HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
[@override](/user/override)
_HomeScreenState createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
[@override](/user/override)
void initState() {
super.initState();
checkForAppUpdate();
}
void checkForAppUpdate() async {
try {
final updateInfo = await InGoogleplayUpdate.checkForUpdate();
if (updateInfo.updateAvailability == UpdateAvailability.updateAvailable) {
showUpdateDialog(updateInfo);
}
} catch (e) {
print("Error checking for update: $e");
}
}
void showUpdateDialog(UpdateInfo updateInfo) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text("New Update Available"),
content: Text("A new version of the app is available. Please update to continue."),
actions: <Widget>[
TextButton(
child: Text("Update"),
onPressed: () {
InGoogleplayUpdate.launchUpdate();
Navigator.of(context).pop();
},
),
TextButton(
child: Text("Later"),
onPressed: () {
Navigator.of(context).pop();
},
),
],
);
},
);
}
[@override](/user/override)
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("App Update Example"),
),
body: Center(
child: Text("Welcome to the app!"),
),
);
}
}