Flutter状态管理插件mobx_triple的使用
Flutter状态管理插件mobx_triple的使用
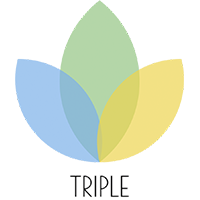
Implementation of the Segmented State Pattern, nicknamed Triple.
文档
特性和问题
The Segmented State Standard is constantly growing.
让我们知道你对这一切的看法。
如果你同意,请给那个代表你签署并同意提议标准的存储库点赞。
问题和建议
issues 频道开放用于提问,报告问题和提出建议,请随时使用此沟通渠道。
让我们一起成为参考
示例代码
示例代码:example/lib/main.dart
// ignore_for_file: invalid_use_of_protected_member
import 'package:flutter/material.dart';
import 'package:mobx_triple/mobx_triple.dart'; // 导入mobx_triple库
import 'counter.dart'; // 导入Counter逻辑文件
void main() {
runApp(MyApp()); // 启动应用程序
}
class MyApp extends StatelessWidget {
// 这个小部件是你的应用的根。
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo', // 应用名称
debugShowCheckedModeBanner: false, // 禁用调试标志
theme: ThemeData( // 主题设置
primarySwatch: Colors.blue,
),
home: MyHomePage(
title: 'Flutter Demo Home Page', // 主页标题
),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key? key, required this.title}) : super(key: key); // 初始化页面
final String title;
@override
_MyHomePageState createState() => _MyHomePageState(); // 创建状态类
}
class _MyHomePageState extends State<MyHomePage> {
final counter = Counter(); // 初始化Counter逻辑实例
// 浮动按钮的状态控制函数
Widget _floatingButton(bool active) {
return FloatingActionButton(
onPressed: active ? counter.increment : null, // 如果活跃则执行increment
tooltip: active ? 'Increment' : 'no-active', // 提示信息
backgroundColor: active ? Theme.of(context).primaryColor : Colors.grey, // 按钮颜色
child: Icon(Icons.add), // 图标
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title), // 标题
actions: [
IconButton(
onPressed: counter.undo, // 撤销操作
icon: Icon(Icons.arrow_back_ios), // 左箭头图标
),
IconButton(
onPressed: counter.redo, // 重做操作
icon: Icon(Icons.arrow_forward_ios), // 右箭头图标
),
],
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center, // 垂直居中
children: <Widget>[
// 加载状态显示
ScopedBuilder(
store: counter,
onLoading: (_) {
return Text(
!counter.isLoading ? 'You have pushed the button this many times:' : 'Carregando...', // 根据加载状态显示文本
);
},
),
// 显示当前计数器值
ScopedBuilder(
store: counter,
onState: (_, int state) {
return Text(
'$state', // 当前计数值
style: Theme.of(context).textTheme.headlineMedium,
);
},
),
],
),
),
floatingActionButton: ScopedBuilder(
store: counter,
onError: (_, error) => _floatingButton(error == null), // 错误状态处理
onLoading: (_) => _floatingButton(!counter.isLoading), // 加载状态处理
onState: (_, __) => _floatingButton(true), // 正常状态处理
),
);
}
}
示例代码:counter.dart
import 'package:mobx/mobx.dart';
import 'package:mobx_triple/mobx_triple.dart';
part 'counter.g.dart';
class Counter with Store, TripleMixin<int> {
[@observable](/user/observable)
int _value = 0;
[@action](/user/action)
void increment() {
if (!_value.isMax()) {
_value++;
setState(_value); // 更新状态
}
}
[@action](/user/action)
void undo() {
if (_value > 0) {
_value--;
setState(_value); // 回退状态
}
}
[@action](/user/action)
void redo() {
if (!_value.isMax()) {
_value++;
setState(_value); // 前进状态
}
}
bool get isLoading => _value.isMax(); // 判断是否达到最大值
}
extension MaxValue on int {
bool get isMax => this >= 10; // 自定义扩展方法判断是否达到最大值
}
更多关于Flutter状态管理插件mobx_triple的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
1 回复