Flutter通知问题诊断插件notification_troubleshoot的使用
Flutter通知问题诊断插件notification_troubleshoot
的使用
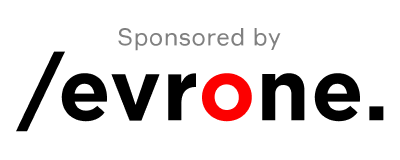
由Evrone.com开发 #
启动特定于供应商的Activity以修复自动启动、省电和通知设置。 了解更多…
对于Android平台,该插件使用了AppKillerManager库。
支持的平台 #
- Android
如果您需要在其他平台上实现任何功能,请创建一个issue。
入门指南 #
您可以获取可用的操作列表:
final List<NotificationTroubleshootActions> availableActions = await NotificationTroubleshoot.availableActions;
在您的应用程序中展示所有可用的操作,并允许用户执行这些操作:
NotificationTroubleshoot.startIntent(availableActions);
插件的目标 #
当使用推送通知时,经常遇到的一个问题是某些Android设备上的通知无法正常工作。这通常是由于制造商添加到Android固件中的自定义服务引起的。例如,省电、阻止推送通知、禁止自动启动等。
一些应用程序通过创建故障排除指南来解决这个问题,比如Slack发布的文章 [https://slack.com/intl/en-ru/help/articles/360001562747-Known-issues-with-Android-notifications]。
然而,在Android设备上有一个名为AppKillerManager的库 [https://github.com/thelittlefireman/AppKillerManager],它可以打开不同供应商的系统设置,让用户允许应用程序在后台运行或将其标记为省电模式下的例外。
当我们使用这个包时,可以在Android版本的应用程序UI中创建“通知问题”项目,并向用户展示他们应该采取的一系列操作,例如:
- “允许应用使用自动启动”
- “移除后台活动限制”
- 其他
现在,该插件解决了Android设备上的问题。如果在其他设备平台(如Web/Desktop)上发现类似问题,请创建一个issue。
示例代码
以下是一个完整的示例代码,展示了如何在Flutter应用中使用notification_troubleshoot
插件。
import 'package:flutter/material.dart';
import 'package:notification_troubleshoot/notification_troubleshoot.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
[@override](/user/override)
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
[@override](/user/override)
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text('插件示例应用'),
),
body: Center(
child: FutureBuilder<List<NotificationTroubleshootActions>>(
/// 获取此设备上的可用操作
future: NotificationTroubleshoot.availableActions,
builder: (context, state) {
var data = state.data;
if (data == null) {
return CircularProgressIndicator();
}
if (data.isEmpty) {
return Text('没有可用的操作');
}
return Column(
mainAxisSize: MainAxisSize.min,
children: [
Text('可用的操作: \n'),
for (final action in data)
ListTile(
title: Text('${action.toString().split('.')[1]}'),
/// 启动特定于供应商的Activity
onTap: () => NotificationTroubleshoot.startIntent(action),
trailing: Icon(
Icons.arrow_forward,
),
)
],
);
},
),
),
),
);
}
}
更多关于Flutter通知问题诊断插件notification_troubleshoot的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
更多关于Flutter通知问题诊断插件notification_troubleshoot的使用的实战系列教程也可以访问 https://www.itying.com/category-92-b0.html
当然,以下是如何在Flutter项目中使用notification_troubleshoot
插件来诊断通知问题的示例代码和步骤。notification_troubleshoot
插件能够帮助你诊断和处理在Flutter应用中遇到的通知问题,比如通知权限、通知通道设置等。
步骤 1: 添加依赖
首先,你需要在pubspec.yaml
文件中添加notification_troubleshoot
依赖:
dependencies:
flutter:
sdk: flutter
notification_troubleshoot: ^x.y.z # 请替换为最新版本号
然后运行flutter pub get
来安装依赖。
步骤 2: 导入插件
在你的Flutter项目中,导入notification_troubleshoot
插件:
import 'package:notification_troubleshoot/notification_troubleshoot.dart';
步骤 3: 使用插件进行诊断
你可以通过调用NotificationTroubleshoot
类的方法来诊断通知问题。以下是一个简单的示例,展示了如何检查通知权限和通道状态:
import 'package:flutter/material.dart';
import 'package:notification_troubleshoot/notification_troubleshoot.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: NotificationDiagnosticsPage(),
);
}
}
class NotificationDiagnosticsPage extends StatefulWidget {
@override
_NotificationDiagnosticsPageState createState() => _NotificationDiagnosticsPageState();
}
class _NotificationDiagnosticsPageState extends State<NotificationDiagnosticsPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Notification Diagnostics'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text('Checking notification permissions and settings...'),
SizedBox(height: 16.0),
ElevatedButton(
onPressed: () async {
// 检查通知权限
bool hasPermission = await NotificationTroubleshoot.checkPermission();
print('Notification Permission: $hasPermission');
// 获取通知通道状态
Map<String, dynamic> notificationChannelsStatus = await NotificationTroubleshoot.getNotificationChannelsStatus();
print('Notification Channels Status: $notificationChannelsStatus');
// 显示结果
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text('Diagnostics Result'),
content: SingleChildScrollView(
child: ListBody(
children: <Widget>[
Text('Permission: $hasPermission'),
SizedBox(height: 16.0),
Text('Channels Status: $notificationChannelsStatus'),
],
),
),
actions: <Widget>[
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text('OK'),
),
],
);
},
);
},
child: Text('Check Notifications'),
),
],
),
),
);
}
}
解释
- 依赖添加:在
pubspec.yaml
中添加notification_troubleshoot
依赖。 - 导入插件:在需要诊断通知问题的Dart文件中导入
notification_troubleshoot
。 - 使用插件:
- 使用
NotificationTroubleshoot.checkPermission()
方法检查通知权限。 - 使用
NotificationTroubleshoot.getNotificationChannelsStatus()
方法获取通知通道的状态。 - 将结果通过对话框显示出来。
- 使用
注意
- 插件的API可能会随着版本的更新而变化,请参考最新的官方文档以获取最准确的API信息。
- 在某些平台上(如iOS),可能需要在
Info.plist
文件中添加特定的权限声明,以确保通知功能正常工作。
通过上述步骤,你可以使用notification_troubleshoot
插件来诊断Flutter应用中的通知问题。